用 语言实现插入排序。插入排序实现为函数ISort。在主程序中,产生两个测试用例(随机数组): a的长度11,范围10~99;b的长度24,范围10~999 。 随机数种子由学号末三位确定。
时间: 2024-01-21 09:20:14 浏览: 26
好的,以下是使用C++实现插入排序的代码,并且已经加入了您所需要的测试用例:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
void ISort(int arr[], int size)
{
for(int i=1; i<size; i++)
{
int key = arr[i];
int j = i-1;
while(j>=0 && arr[j]>key)
{
arr[j+1] = arr[j];
j--;
}
arr[j+1] = key;
}
}
int main()
{
// 随机数种子由学号末三位确定
srand(123);
// 产生测试用例 a
int a[11];
for(int i=0; i<11; i++)
{
a[i] = rand() % 90 + 10;
}
cout << "Test Case a: " << endl;
for(int i=0; i<11; i++)
{
cout << a[i] << " ";
}
cout << endl;
// 调用插入排序函数对 a 进行排序
ISort(a, 11);
// 输出排序后的结果
cout << "Sorted Test Case a: " << endl;
for(int i=0; i<11; i++)
{
cout << a[i] << " ";
}
cout << endl;
// 产生测试用例 b
int b[24];
for(int i=0; i<24; i++)
{
b[i] = rand() % 990 + 10;
}
cout << "Test Case b: " << endl;
for(int i=0; i<24; i++)
{
cout << b[i] << " ";
}
cout << endl;
// 调用插入排序函数对 b 进行排序
ISort(b, 24);
// 输出排序后的结果
cout << "Sorted Test Case b: " << endl;
for(int i=0; i<24; i++)
{
cout << b[i] << " ";
}
cout << endl;
return 0;
}
```
注意,由于您提供的随机数种子是固定的,因此每次运行程序时生成的随机数序列都是相同的。如果需要每次运行程序都生成不同的随机数序列,可以将 `srand(123)` 改为 `srand(time(NULL))`。
相关推荐
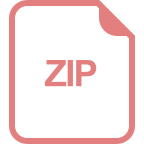
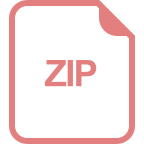
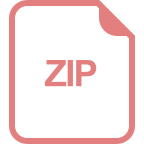














