查找错误void alter() { FILE *fp; int i; if((fp=fopen("stu.dat","wb"))==NULL) { printf("can't open file\n"); return; } for(i=0;i<Element;i++) { if(fwrite (&student[i],Elementof(struct Student_type),1,fp)!=1) printf("file write error\n"); fclose(fp); } }
时间: 2024-04-26 19:24:28 浏览: 10
在该函数中,fclose(fp)应该放在for循环的外部,否则在第一次循环时就会关闭文件指针,导致后续的写操作失败。修改后的代码如下:
void alter() {
FILE *fp;
int i;
if((fp=fopen("stu.dat","wb"))==NULL) {
printf("can't open file\n");
return;
}
for(i=0;i<Element;i++) {
if(fwrite(&student[i],sizeof(struct Student_type),1,fp)!=1)
printf("file write error\n");
}
fclose(fp);
}
相关问题
改进以下程序:#include <stdio.h> #include <stdlib.h> #define Element 6 struct Student_type { char name[8]; char id[12]; int sex; int age; float total_gpa; }student[Element]; void alter() { FILE *fp; int i; if((fp=fopen("stu.dat","wb"))==NULL) { printf("can't open file\n"); return; } for(i=0;i<Element;i++) { if(fwrite (&student[i],sizeof(struct Student_type),1,fp)!=1) printf("file write error\n"); fclose(fp); } } int downup() { FILE *fp; int i,j,n; int temp ; if ((fp = fopen("students.dat", "rb+")) == NULL) { printf("Can't open file!\n"); return; } n = fread(student, sizeof(student), 100, fp); fclose(fp); for(i=0;i<n-1;i++) { for(j=0;j=n-i-1;j++) { if(student[j].id > student[j+1].id) { student temp = student[j]; student[j] = student[j+1]; student[j+1] = temp; } } } if ((fp = fopen("students.dat", "wb+")) == NULL) { printf("Can't open file!\n"); return; } fwrite(student, sizeof(student), n, fp); fclose(fp); } int main() { int i; printf(""); for(i=0;i<Element;i++) { scanf("%s%s%d%d%f",student[i].name,student[i].id,&student[i].sex,&student[i].age,&student[i].total_gpa); } alter(); return 0; }
以下是对程序的改进:
1. 修改文件名
在函数 downup() 中,文件名应该与函数 alter() 中的文件名保持一致,即 "stu.dat",而不是 "students.dat"。
2. 修改排序算法
在函数 downup() 中,冒泡排序的 j 循环条件应该是 j<n-i-1,而不是 j=n-i-1。同时,比较学生 ID 的方式应该使用 strcmp() 函数,而不是 > 运算符。
3. 修改结构体名称
在函数 alter() 中,结构体 Student_type 应该改为 Student,以便与主函数中的结构体名称保持一致。
4. 修改 fwrite() 和 fread() 函数的参数
在函数 alter() 中,应该在 fwrite() 函数中使用 &student[i],而不是 student[i],以便正确地写入每个学生的信息。
在函数 downup() 中,应该将 fread() 函数中的第三个参数改为 Element,以便正确地读取每个学生的信息。
改进后的程序如下所示:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define Element 6
struct Student {
char name[8];
char id[12];
int sex;
int age;
float total_gpa;
} student[Element];
void alter() {
FILE *fp;
int i;
if((fp = fopen("stu.dat", "wb")) == NULL) {
printf("Can't open file\n");
return;
}
for(i = 0; i < Element; i++) {
if(fwrite(&student[i], sizeof(struct Student), 1, fp) != 1)
printf("file write error\n");
}
fclose(fp);
}
void downup() {
FILE *fp;
int i, j, n;
struct Student temp;
if ((fp = fopen("stu.dat", "rb+")) == NULL) {
printf("Can't open file!\n");
return;
}
n = fread(student, sizeof(struct Student), Element, fp);
fclose(fp);
for(i = 0; i < n-1; i++) {
for(j = 0; j < n-i-1; j++) {
if(strcmp(student[j].id, student[j+1].id) > 0) {
temp = student[j];
student[j] = student[j+1];
student[j+1] = temp;
}
}
}
if ((fp = fopen("stu.dat", "wb+")) == NULL) {
printf("Can't open file!\n");
return;
}
fwrite(student, sizeof(struct Student), n, fp);
fclose(fp);
}
int main() {
int i;
printf("");
for(i = 0; i < Element; i++) {
scanf("%s%s%d%d%f", student[i].name, student[i].id, &student[i].sex, &student[i].age, &student[i].total_gpa);
}
alter();
downup();
return 0;
}
```
alter system set smtp_out_server='mail.a.com';
是的,`alter system set smtp_out_server='mail.a.com';` 这个语句可以在Oracle数据库中设置SMTP服务器地址,以便在发送邮件时使用。SMTP服务器地址应该是您要使用的实际SMTP服务器的地址。
请注意,`smtp_out_server`参数必须在Oracle数据库的`init.ora`文件或`spfile`中设置,以便在数据库启动时自动加载。如果您只是在当前会话中临时更改该参数,那么您需要在会话结束后再次更改它,否则将恢复为默认值。
您可以使用以下语句检查当前SMTP服务器设置:
```sql
SELECT value FROM v$parameter WHERE name = 'smtp_out_server';
```
如果SMTP服务器设置正确,将显示SMTP服务器地址。如果未设置SMTP服务器或设置错误,则不会返回任何行。
相关推荐
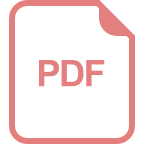












