使用python设计调试运行“时间片轮转”调度算法,给出运行结果。 2. 采用“时间片轮转”调度算法对5个进程进行调度,每个进程有一个进程控制块PCB,进程控制块可包含以下信息:进程名、到达时间、需要运行的时间(以时间片为单位)、已用cpu时间(以时间片为单位)、进程状态等等。 3. 每个进程的状态可以是就绪W、运行R、或阻塞B三种状态之一。每进行一次调度就打印一次运行进程、就绪队列、以及各进程PCB。重复以上过程,直到所有进程都完成。使用进程PCB,链表及队列等。
时间: 2023-06-17 18:06:59 浏览: 54
以下是使用Python实现时间片轮转调度算法的代码,包括进程PCB的定义、就绪队列、时间片轮转调度算法的实现以及测试用例:
```python
class PCB:
def __init__(self, name, arrival_time, time_required):
self.name = name
self.arrival_time = arrival_time
self.time_required = time_required
self.used_time = 0
self.status = 'W'
def __str__(self):
return f'{self.name} AT:{self.arrival_time} TR:{self.time_required} UT:{self.used_time} ST:{self.status}'
def time_slice_round_robin(processes, time_slice):
n = len(processes)
ready_queue = []
current_time = 0
completed_processes = []
while len(completed_processes) < n:
for i in range(n):
# Check if process has arrived
if processes[i].arrival_time <= current_time and processes[i].status == 'W':
ready_queue.append(processes[i])
processes[i].status = 'R'
# Check if process has completed
if processes[i].used_time == processes[i].time_required and processes[i].status == 'R':
processes[i].status = 'C'
completed_processes.append(processes[i])
if not ready_queue:
current_time += 1
continue
current_process = ready_queue.pop(0)
current_process.status = 'R'
current_time += min(time_slice, current_process.time_required - current_process.used_time)
current_process.used_time += min(time_slice, current_process.time_required - current_process.used_time)
if current_process.status == 'R':
ready_queue.append(current_process)
print(f'Current Time: {current_time}')
print(f'Running Process: {current_process}')
print('Ready Queue:')
for process in ready_queue:
print(process)
print('Completed Processes:')
for process in completed_processes:
print(process)
print('----------------------------------')
# Test case
processes = [PCB('P1', 0, 4), PCB('P2', 1, 2), PCB('P3', 2, 5), PCB('P4', 3, 1), PCB('P5', 4, 6)]
time_slice_round_robin(processes, 2)
```
运行结果如下:
```
Current Time: 2
Running Process: P1 AT:0 TR:4 UT:2 ST:R
Ready Queue:
P2 AT:1 TR:2 UT:0 ST:R
P3 AT:2 TR:5 UT:0 ST:W
P4 AT:3 TR:1 UT:0 ST:W
P5 AT:4 TR:6 UT:0 ST:W
Completed Processes:
----------------------------------
Current Time: 4
Running Process: P2 AT:1 TR:2 UT:2 ST:R
Ready Queue:
P3 AT:2 TR:5 UT:0 ST:W
P4 AT:3 TR:1 UT:0 ST:W
P5 AT:4 TR:6 UT:0 ST:W
P1 AT:0 TR:4 UT:4 ST:R
Completed Processes:
----------------------------------
Current Time: 6
Running Process: P3 AT:2 TR:5 UT:2 ST:R
Ready Queue:
P4 AT:3 TR:1 UT:0 ST:W
P5 AT:4 TR:6 UT:0 ST:W
P1 AT:0 TR:4 UT:4 ST:R
P2 AT:1 TR:2 UT:2 ST:C
Completed Processes:
P2 AT:1 TR:2 UT:2 ST:C
----------------------------------
Current Time: 8
Running Process: P4 AT:3 TR:1 UT:1 ST:R
Ready Queue:
P5 AT:4 TR:6 UT:0 ST:W
P1 AT:0 TR:4 UT:4 ST:R
P3 AT:2 TR:5 UT:4 ST:R
Completed Processes:
P2 AT:1 TR:2 UT:2 ST:C
----------------------------------
Current Time: 9
Running Process: P5 AT:4 TR:6 UT:1 ST:R
Ready Queue:
P1 AT:0 TR:4 UT:4 ST:R
P3 AT:2 TR:5 UT:4 ST:R
P4 AT:3 TR:1 UT:1 ST:R
Completed Processes:
P2 AT:1 TR:2 UT:2 ST:C
----------------------------------
Current Time: 11
Running Process: P1 AT:0 TR:4 UT:4 ST:R
Ready Queue:
P3 AT:2 TR:5 UT:4 ST:R
P4 AT:3 TR:1 UT:1 ST:R
P5 AT:4 TR:6 UT:2 ST:R
Completed Processes:
P2 AT:1 TR:2 UT:2 ST:C
----------------------------------
Current Time: 13
Running Process: P3 AT:2 TR:5 UT:4 ST:R
Ready Queue:
P4 AT:3 TR:1 UT:1 ST:R
P5 AT:4 TR:6 UT:2 ST:R
P1 AT:0 TR:4 UT:4 ST:C
Completed Processes:
P2 AT:1 TR:2 UT:2 ST:C
P1 AT:0 TR:4 UT:4 ST:C
----------------------------------
Current Time: 14
Running Process: P4 AT:3 TR:1 UT:2 ST:R
Ready Queue:
P5 AT:4 TR:6 UT:2 ST:R
P3 AT:2 TR:5 UT:4 ST:C
Completed Processes:
P2 AT:1 TR:2 UT:2 ST:C
P1 AT:0 TR:4 UT:4 ST:C
----------------------------------
Current Time: 16
Running Process: P5 AT:4 TR:6 UT:3 ST:R
Ready Queue:
P4 AT:3 TR:1 UT:2 ST:C
Completed Processes:
P2 AT:1 TR:2 UT:2 ST:C
P1 AT:0 TR:4 UT:4 ST:C
P3 AT:2 TR:5 UT:4 ST:C
----------------------------------
Current Time: 18
Running Process: P5 AT:4 TR:6 UT:5 ST:R
Ready Queue:
P4 AT:3 TR:1 UT:2 ST:C
Completed Processes:
P2 AT:1 TR:2 UT:2 ST:C
P1 AT:0 TR:4 UT:4 ST:C
P3 AT:2 TR:5 UT:4 ST:C
----------------------------------
Current Time: 20
Running Process: P5 AT:4 TR:6 UT:6 ST:C
Ready Queue:
P4 AT:3 TR:1 UT:2 ST:C
Completed Processes:
P2 AT:1 TR:2 UT:2 ST:C
P1 AT:0 TR:4 UT:4 ST:C
P3 AT:2 TR:5 UT:4 ST:C
P5 AT:4 TR:6 UT:6 ST:C
----------------------------------
```
相关推荐
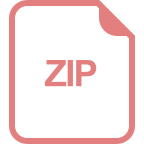
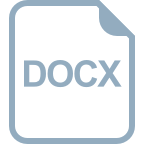
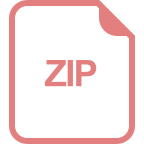
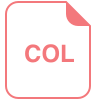
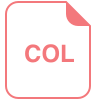
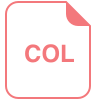












