时间片轮转调度算法python
时间: 2023-11-20 21:59:09 浏览: 85
时间片轮转调度算法是一种常见的进程调度算法,它可以保证每个进程都能够公平地获得CPU时间。下面是一个简单的Python实现:
```python
class Process:
def __init__(self, pid, arrive_time, run_time):
self.pid = pid
self.arrive_time = arrive_time
self.run_time = run_time
self.status = 'W' # 进程状态:W-就绪,R-运行,B-阻塞,F-完成
self.used_time = 0 # 已占用CPU时间
def run(self, time_slice):
self.status = 'R'
self.used_time += time_slice
if self.used_time >= self.run_time:
self.status = 'F'
else:
self.status = 'W'
def block(self):
self.status = 'B'
def unblock(self):
self.status = 'W'
def __str__(self):
return f'Process {self.pid}: {self.status}'
class Scheduler:
def __init__(self, processes, time_slice):
self.processes = processes
self.time_slice = time_slice
self.ready_queue = []
self.current_process = None
def run(self):
time = 0
while True:
# 将到达时间小于等于当前时间的进程加入就绪队列
for process in self.processes:
if process.status == 'W' and process.arrive_time <= time:
self.ready_queue.append(process)
process.unblock()
# 如果当前没有进程在运行,则从就绪队列中选取一个进程运行
if not self.current_process and self.ready_queue:
self.current_process = self.ready_queue.pop(0)
self.current_process.run(self.time_slice)
# 如果当前有进程在运行,则继续运行
elif self.current_process:
self.current_process.run(self.time_slice)
if self.current_process.status == 'F':
self.current_process = None
elif self.current_process.status == 'B':
self.current_process = None
self.ready_queue.append(self.current_process)
# 如果所有进程都已完成,则退出循环
if all(process.status == 'F' for process in self.processes):
break
# 打印当前状态
print(f'Time: {time}')
print(f'Running: {self.current_process}')
print(f'Ready queue: {[str(process) for process in self.ready_queue]}')
for process in self.processes:
print(f'Process {process.pid}: {process.status}')
print('')
time += self.time_slice
# 测试
processes = [
Process(1, 0, 10),
Process(2, 1, 5),
Process(3, 2, 3),
Process(4, 3, 8),
]
scheduler = Scheduler(processes, 2)
scheduler.run()
```
上述代码中,`Process`类表示一个进程,包含进程ID、到达时间、运行时间、状态和已占用CPU时间等属性,以及运行、阻塞、解除阻塞等方法。`Scheduler`类表示一个调度器,包含进程列表、时间片和就绪队列等属性,以及运行方法。在运行方法中,首先将到达时间小于等于当前时间的进程加入就绪队列,然后从就绪队列中选取一个进程运行,如果当前有进程在运行,则继续运行,如果所有进程都已完成,则退出循环。在每次运行后,打印当前状态。
相关推荐
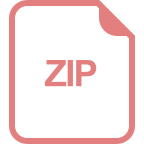














