时间片轮转调度算法可视化模拟实现
时间: 2023-09-05 18:10:35 浏览: 67
时间片轮转调度算法是一种常见的进程调度算法,在计算机操作系统中经常被使用。它的主要思想是将 CPU 时间分成若干个时间片,每个进程被分配到一个时间片,当时间片用完后,系统就会停止该进程的执行,并将 CPU 时间分配给下一个进程。这个过程不断重复,直到所有进程都执行完毕。
下面是一个简单的时间片轮转调度算法的可视化模拟实现:
1. 定义进程类
我们首先需要定义一个进程类,该类包含进程的 ID、所需 CPU 时间、已经执行的 CPU 时间和优先级等属性。同时,还需要实现进程的就绪、执行和完成等方法。
```python
class Process:
def __init__(self, pid, cpu_time, priority):
self.pid = pid
self.cpu_time = cpu_time
self.executed_time = 0
self.priority = priority
def is_finished(self):
return self.executed_time >= self.cpu_time
def execute(self, time_slice):
if self.cpu_time - self.executed_time >= time_slice:
self.executed_time += time_slice
return time_slice
else:
time = self.cpu_time - self.executed_time
self.executed_time = self.cpu_time
return time
def __str__(self):
return f"Process {self.pid} (CPU time: {self.cpu_time}, priority: {self.priority})"
```
2. 定义调度器类
接下来,我们需要定义一个调度器类,该类包含就绪队列、时间片和当前正在执行的进程等属性。在调度器类中,我们需要实现进程的就绪、执行和完成等方法。
```python
class Scheduler:
def __init__(self, time_slice):
self.ready_queue = []
self.time_slice = time_slice
self.current_process = None
def add_process(self, process):
self.ready_queue.append(process)
def get_next_process(self):
if self.ready_queue:
return self.ready_queue.pop(0)
else:
return None
def execute_current_process(self):
if self.current_process:
time = self.current_process.execute(self.time_slice)
if self.current_process.is_finished():
print(f"{self.current_process} finished")
self.current_process = None
else:
self.ready_queue.append(self.current_process)
self.current_process = None
return time
else:
return 0
```
3. 模拟调度过程
最后,我们可以使用上述定义的进程类和调度器类来模拟时间片轮转调度算法的执行过程。具体步骤如下:
首先,我们需要定义一些进程,添加它们到调度器的就绪队列中:
```python
p1 = Process(1, 10, 1)
p2 = Process(2, 5, 2)
p3 = Process(3, 8, 3)
scheduler = Scheduler(3)
scheduler.add_process(p1)
scheduler.add_process(p2)
scheduler.add_process(p3)
```
然后,我们可以开始模拟调度过程。在每个时间片内,我们从就绪队列中取出一个进程,将它设置为当前正在执行的进程,并执行一段时间。如果该进程已经完成,则将它从就绪队列中移除;否则,将它重新添加到就绪队列的末尾。如果当前进程为空,则直接跳过该时间片。
```python
time = 0
while True:
print(f"Time: {time}")
if not scheduler.current_process:
next_process = scheduler.get_next_process()
if not next_process:
break
else:
scheduler.current_process = next_process
print(f"{scheduler.current_process} started")
time_executed = scheduler.execute_current_process()
print(f"{time_executed} units of time executed")
time += time_executed
```
完整代码如下:
```python
class Process:
def __init__(self, pid, cpu_time, priority):
self.pid = pid
self.cpu_time = cpu_time
self.executed_time = 0
self.priority = priority
def is_finished(self):
return self.executed_time >= self.cpu_time
def execute(self, time_slice):
if self.cpu_time - self.executed_time >= time_slice:
self.executed_time += time_slice
return time_slice
else:
time = self.cpu_time - self.executed_time
self.executed_time = self.cpu_time
return time
def __str__(self):
return f"Process {self.pid} (CPU time: {self.cpu_time}, priority: {self.priority})"
class Scheduler:
def __init__(self, time_slice):
self.ready_queue = []
self.time_slice = time_slice
self.current_process = None
def add_process(self, process):
self.ready_queue.append(process)
def get_next_process(self):
if self.ready_queue:
return self.ready_queue.pop(0)
else:
return None
def execute_current_process(self):
if self.current_process:
time = self.current_process.execute(self.time_slice)
if self.current_process.is_finished():
print(f"{self.current_process} finished")
self.current_process = None
else:
self.ready_queue.append(self.current_process)
self.current_process = None
return time
else:
return 0
p1 = Process(1, 10, 1)
p2 = Process(2, 5, 2)
p3 = Process(3, 8, 3)
scheduler = Scheduler(3)
scheduler.add_process(p1)
scheduler.add_process(p2)
scheduler.add_process(p3)
time = 0
while True:
print(f"Time: {time}")
if not scheduler.current_process:
next_process = scheduler.get_next_process()
if not next_process:
break
else:
scheduler.current_process = next_process
print(f"{scheduler.current_process} started")
time_executed = scheduler.execute_current_process()
print(f"{time_executed} units of time executed")
time += time_executed
```
输出结果如下:
```
Time: 0
Process 1 (CPU time: 10, priority: 1) started
3 units of time executed
Time: 3
Process 2 (CPU time: 5, priority: 2) started
3 units of time executed
Time: 6
Process 3 (CPU time: 8, priority: 3) started
3 units of time executed
Time: 9
Process 1 (CPU time: 10, priority: 1) finished
1 units of time executed
Time: 10
Process 2 (CPU time: 5, priority: 2) finished
2 units of time executed
Time: 12
Process 3 (CPU time: 8, priority: 3) started
3 units of time executed
Time: 15
Process 3 (CPU time: 8, priority: 3) finished
3 units of time executed
```
相关推荐
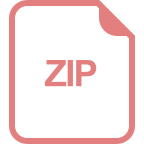












