执行webdriver.ActionChains(driver).move_to_element(element).click(element).perform()时,报错selenium.common.exceptions.JavascriptException: Message: javascript error: Failed to execute 'elementsFromPoint' on 'Document': The provided double value is non-finite.这是为什么?
时间: 2024-03-20 17:41:03 浏览: 23
这个错误通常是由于 `element` 定位到的元素不在当前的可视区域内,导致 `move_to_element` 方法无法正确执行。在这种情况下,`click` 操作可能会被视为非法的,并导致 JavaScript 错误。
为了解决这个问题,你可以尝试使用 `WebDriverWait` 显式等待,直到元素可见后再执行操作,例如:
```python
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, "my-element"))
)
actions = webdriver.ActionChains(driver)
actions.move_to_element(element).click().perform()
```
这个方法首先使用 `WebDriverWait` 等待元素可见,然后使用 `ActionChains` 执行操作。
如果你还是遇到问题,请提供完整的错误信息和代码,以便更好地定位问题。
相关问题
from selenium import webdriver import time from selenium.webdriver.chrome.service import Service from selenium.webdriver.common.by import By from selenium.webdriver.common.action_chains import ActionChains # 读取操作excel表格 import openpyxl , 上述代码如何进行封装, 方便引用
可以将上述代码封装成一个类,如下所示:
```
import openpyxl
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.common.action_chains import ActionChains
class Login:
def __init__(self, username, password):
self.username = username
self.password = password
self.wb = openpyxl.load_workbook('example.xlsx')
self.ws = self.wb.active
self.service = Service('chromedriver')
self.driver = webdriver.Chrome(service=self.service)
def login(self):
self.driver.get('https://www.example.com/login')
# 输入用户名和密码
username_field = self.driver.find_element(By.ID, 'username')
password_field = self.driver.find_element(By.ID, 'password')
username_field.send_keys(self.username)
password_field.send_keys(self.password)
# 点击登录按钮
login_button = self.driver.find_element(By.ID, 'login-button')
ActionChains(self.driver).move_to_element(login_button).click().perform()
def logout(self):
# 退出登录
logout_button = self.driver.find_element(By.ID, 'logout-button')
ActionChains(self.driver).move_to_element(logout_button).click().perform()
self.driver.quit()
self.wb.save('example.xlsx')
```
然后在其他文件中可以实例化该类并调用其中的方法,如下所示:
```
from login import Login
# 实例化 Login 类并登录账号
login = Login('your_username', 'your_password')
login.login()
# 在需要退出登录的地方调用 logout 方法
login.logout()
```
这样可以将登录和退出登录的代码封装在一个类中,便于管理和维护。
selenium move_to_element问题
`move_to_element()` 是 Selenium WebDriver 中的一个方法,用于将鼠标移动到指定元素的位置。其语法如下:
```
move_to_element(to_element)
```
其中,`to_element` 表示要移动到的元素对象。例如,要将鼠标移动到页面上的某个元素,可以使用以下代码:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
# 创建 WebDriver 对象
driver = webdriver.Chrome()
# 打开网页
driver.get('https://www.example.com')
# 定位要移动到的元素
element = driver.find_element_by_xpath('//a[@class="example"]')
# 创建 ActionChains 对象
actions = ActionChains(driver)
# 将鼠标移动到元素位置
actions.move_to_element(element).perform()
```
在上面的示例中,首先使用 `driver.find_element_by_xpath` 方法定位到要移动到的元素,然后使用 `ActionChains` 类创建对象。接着,使用 `move_to_element()` 方法将鼠标移动到元素位置,最后使用 `perform()` 方法执行操作。
需要注意的是,`move_to_element()` 方法只是将鼠标移动到指定元素的位置,并不会触发任何事件。如果需要在元素上执行其他操作,可以在移动到元素后继续使用 `ActionChains` 类的其他方法,如 `click()`、`context_click()` 等。
相关推荐
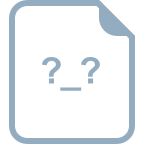
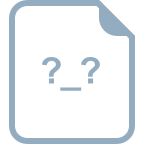
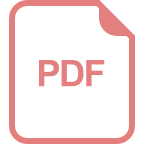












