java中如何通过ftp连接获取所有文件内容
时间: 2024-02-06 07:04:43 浏览: 148
可以使用Apache Commons Net库来连接FTP服务器并获取所有文件内容。下面是一个简单的Java代码示例:
```java
import java.io.IOException;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPFile;
public class FTPExample {
public static void main(String[] args) {
FTPClient ftp = new FTPClient();
try {
ftp.connect("ftp.example.com");
ftp.login("username", "password");
ftp.enterLocalPassiveMode();
ftp.setFileType(FTP.BINARY_FILE_TYPE);
FTPFile[] files = ftp.listFiles();
for (FTPFile file : files) {
if (!file.isFile()) {
continue;
}
String content = readFileContent(ftp, file.getName());
System.out.println("File: " + file.getName() + ", Content: " + content);
}
ftp.logout();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (ftp.isConnected()) {
try {
ftp.disconnect();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
private static String readFileContent(FTPClient ftp, String filename) throws IOException {
StringBuilder sb = new StringBuilder();
ftp.retrieveFile(filename, inputStream -> {
int c;
while ((c = inputStream.read()) != -1) {
sb.append((char) c);
}
});
return sb.toString();
}
}
```
在上面的示例中,我们首先连接到FTP服务器,然后通过`ftp.listFiles()`方法获取所有文件列表。对于每个文件,我们调用`readFileContent()`方法来获取其内容。在`readFileContent()`方法中,我们使用`ftp.retrieveFile()`方法来下载文件并读取其内容。
阅读全文
相关推荐






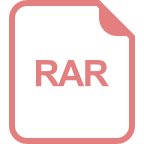





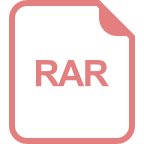




