Java使用FTP获取文件流
时间: 2024-04-12 12:28:53 浏览: 179
要使用 Java 从 FTP 获取文件流,你可以使用`FTPClient`类中的`retrieveFileStream`方法。以下是一个示例代码,演示如何获取文件流:
```java
import org.apache.commons.net.ftp.FTPClient;
import java.io.*;
public class FTPExample {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String username = "your-username";
String password = "your-password";
String remoteFilePath = "/path/to/file.txt";
String localFilePath = "/path/to/save/file.txt";
FTPClient ftpClient = new FTPClient();
try {
// 连接FTP服务器
ftpClient.connect(server, port);
ftpClient.login(username, password);
// 获取文件流
InputStream inputStream = ftpClient.retrieveFileStream(remoteFilePath);
// 将文件流保存到本地文件
File file = new File(localFilePath);
OutputStream outputStream = new FileOutputStream(file);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
// 关闭流
inputStream.close();
outputStream.close();
// 完成文件下载后,调用 completePendingCommand 方法
boolean success = ftpClient.completePendingCommand();
if (success) {
System.out.println("File downloaded successfully.");
} else {
System.out.println("File download failed.");
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
// 断开连接
ftpClient.disconnect();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
在上述代码中,你需要替换 `server`、`port`、`username`、`password`、`remoteFilePath` 和 `localFilePath` 为你实际的 FTP 服务器和文件路径信息。然后使用 `ftpClient.retrieveFileStream(remoteFilePath)` 方法获取文件流,将文件流写入本地文件。
请注意,获取文件流后,你还需要调用 `completePendingCommand` 方法来完成文件传输。这将告诉 FTP 服务器该文件已经接收完毕。
希望这可以帮助到你!如有更多问题,请随时提问。
阅读全文
相关推荐
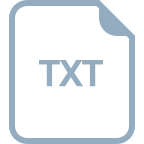


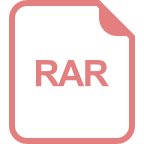
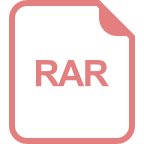
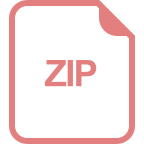
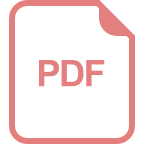
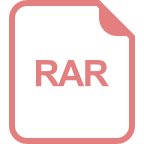
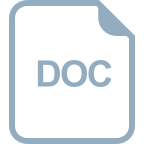
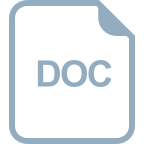






