编写生产者消费者问题的标准C语言代码
时间: 2023-10-21 21:10:59 浏览: 98
以下是一个简单的生产者消费者问题的标准C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 5 // 缓冲区大小
#define PRODUCER_NUM 3 // 生产者数量
#define CONSUMER_NUM 2 // 消费者数量
#define ITEM_NUM 20 // 生产的总数
int buffer[BUFFER_SIZE]; // 缓冲区数组
int in = 0; // 生产者放置产品的位置
int out = 0; // 消费者取产品的位置
int count = 0; // 缓冲区中的产品数目
void *producer(void *arg) // 生产者线程函数
{
int i, item;
for (i = 0; i < ITEM_NUM; i++) {
item = rand() % 1000; // 生产一个随机数
while (count == BUFFER_SIZE) ; // 缓冲区已满,等待
buffer[in] = item; // 放入一个产品
in = (in + 1) % BUFFER_SIZE; // 放置位置后移
count++; // 产品数目加1
printf("Producer %d produce item %d\n", (int)arg, item);
}
pthread_exit(NULL);
}
void *consumer(void *arg) // 消费者线程函数
{
int i, item;
for (i = 0; i < ITEM_NUM / CONSUMER_NUM; i++) {
while (count == 0) ; // 缓冲区为空,等待
item = buffer[out]; // 取出一个产品
out = (out + 1) % BUFFER_SIZE; // 取出位置后移
count--; // 产品数目减1
printf("Consumer %d consume item %d\n", (int)arg, item);
}
pthread_exit(NULL);
}
int main()
{
int i;
pthread_t producer_thread[PRODUCER_NUM];
pthread_t consumer_thread[CONSUMER_NUM];
srand(time(NULL)); // 随机数种子初始化
// 创建生产者线程
for (i = 0; i < PRODUCER_NUM; i++)
pthread_create(&producer_thread[i], NULL, producer, (void*)i);
// 创建消费者线程
for (i = 0; i < CONSUMER_NUM; i++)
pthread_create(&consumer_thread[i], NULL, consumer, (void*)i);
// 等待生产者线程结束
for (i = 0; i < PRODUCER_NUM; i++)
pthread_join(producer_thread[i], NULL);
// 等待消费者线程结束
for (i = 0; i < CONSUMER_NUM; i++)
pthread_join(consumer_thread[i], NULL);
return 0;
}
```
上述代码创建了3个生产者线程和2个消费者线程,他们共同访问一个大小为5的缓冲区,生产者随机生成一个数并放入缓冲区中,消费者从缓冲区中取出一个数并输出。在这个过程中,为了防止生产者在缓冲区已满时一直占用CPU,消费者在缓冲区为空时一直占用CPU,使用了while循环进行等待。
阅读全文
相关推荐
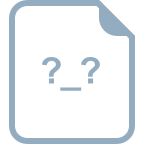

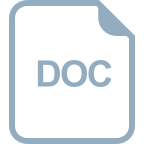









