基于vscode人脸识别考勤系统代码用http和python
时间: 2023-08-21 16:03:41 浏览: 56
以下是一个基于Python语言、OpenCV、face_recognition库和Flask框架的人脸识别考勤系统代码示例,可以通过HTTP协议实现远程访问,可以在vscode中运行:
```python
import cv2
import face_recognition
import os
import datetime
from flask import Flask, jsonify, request
# 加载已知员工的人脸图像和姓名
known_face_encodings = []
known_face_names = []
for file in os.listdir('known_faces'):
if file.endswith('.jpg') or file.endswith('.jpeg') or file.endswith('.png'):
image = face_recognition.load_image_file(os.path.join('known_faces', file))
face_encoding = face_recognition.face_encodings(image)[0]
known_face_encodings.append(face_encoding)
known_face_names.append(os.path.splitext(file)[0])
# 初始化Flask应用
app = Flask(__name__)
# 初始化一些变量
face_locations = []
face_encodings = []
face_names = []
attendance = {}
# 定义考勤接口
@app.route('/attendance', methods=['POST'])
def attendance():
# 读取图像
image = request.files['image'].read()
# 将图像从字节流转换为OpenCV图像格式
nparr = np.fromstring(image, np.uint8)
frame = cv2.imdecode(nparr, cv2.IMREAD_COLOR)
# 缩小图像以加快人脸识别速度
small_frame = cv2.resize(frame, (0, 0), fx=0.25, fy=0.25)
# 将图像从BGR颜色空间转换为RGB颜色空间
rgb_small_frame = small_frame[:, :, ::-1]
# 检测当前帧中的所有人脸
face_locations = face_recognition.face_locations(rgb_small_frame)
face_encodings = face_recognition.face_encodings(rgb_small_frame, face_locations)
face_names = []
for face_encoding in face_encodings:
# 将当前人脸与已知员工的人脸进行比较
matches = face_recognition.compare_faces(known_face_encodings, face_encoding)
name = "Unknown"
# 如果识别出当前人脸属于已知员工,则将其姓名记录在attendance字典中
if True in matches:
first_match_index = matches.index(True)
name = known_face_names[first_match_index]
attendance[name] = datetime.datetime.now().strftime("%Y-%m-%d %H:%M:%S")
face_names.append(name)
# 在图像上绘制人脸边框和姓名
for (top, right, bottom, left), name in zip(face_locations, face_names):
# 放大边框以匹配缩小的图像
top *= 4
right *= 4
bottom *= 4
left *= 4
# 在图像上绘制人脸边框
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
# 在图像上绘制姓名
cv2.rectangle(frame, (left, bottom - 35), (right, bottom), (0, 0, 255), cv2.FILLED)
font = cv2.FONT_HERSHEY_DUPLEX
cv2.putText(frame, name, (left + 6, bottom - 6), font, 1.0, (255, 255, 255), 1)
# 返回带有考勤记录的JSON响应
return jsonify(attendance)
if __name__ == '__main__':
# 启动Flask应用
app.run(host='0.0.0.0')
```
这个示例代码的工作原理如下:
1. 加载已知员工的人脸图像和姓名,并对其进行编码。
2. 初始化Flask应用。
3. 定义一个考勤接口,用于接收一个图像,在其中检测人脸并将考勤记录返回为JSON响应。
4. 在考勤接口中,读取图像并将其从字节流转换为OpenCV图像格式。
5. 对当前帧中的所有人脸进行检测和编码。
6. 将当前人脸与已知员工的人脸进行比较,如果识别出当前人脸属于已知员工,则将其姓名记录在attendance字典中。
7. 在图像上绘制人脸边框和姓名。
8. 返回带有考勤记录的JSON响应。
请注意,这只是一个简单的示例,您需要根据您的实际需求进行修改和扩展。另外,由于本示例代码中的人脸识别模型是在每个HTTP请求中重新加载的,因此可能会导致响应时间较长。您可以考虑将模型加载到内存中并使用多线程或多进程来处理请求,以提高性能。
相关推荐
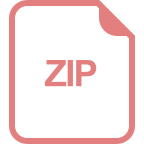
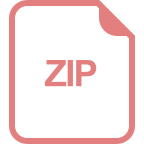
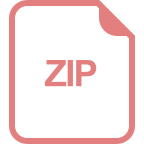














