c++ curl har file
时间: 2023-09-25 08:14:10 浏览: 164
你可以使用C++结合Curl库来读取和解析HAR文件。HAR(HTTP Archive)是一种记录网络请求和响应的标准格式。
首先,你需要使用Curl库来发送网络请求并获取响应。Curl库提供了丰富的API来处理HTTP请求和响应。
以下是一个简单的示例代码,展示了如何使用Curl库读取HAR文件并发送相应的HTTP请求:
```cpp
#include <iostream>
#include <fstream>
#include <curl/curl.h>
// 回调函数,用于接收Curl库的HTTP响应
size_t write_callback(char* ptr, size_t size, size_t nmemb, std::string* response) {
size_t totalSize = size * nmemb;
response->append(ptr, totalSize);
return totalSize;
}
int main() {
// 读取HAR文件
std::ifstream harFile("example.har");
if (!harFile) {
std::cout << "Failed to open HAR file." << std::endl;
return 1;
}
// 解析HAR文件,获取URL
std::string url;
std::string line;
while (std::getline(harFile, line)) {
if (line.find("\"url\":") != std::string::npos) {
size_t startPos = line.find(":") + 2;
size_t endPos = line.find("\",");
url = line.substr(startPos, endPos - startPos);
break;
}
}
harFile.close();
// 使用Curl库发送HTTP请求
CURL* curl = curl_easy_init();
if (curl) {
std::string response;
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_callback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &response);
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
std::cout << "Failed to send HTTP request: " << curl_easy_strerror(res) << std::endl;
}
else {
std::cout << "Response:\n" << response << std::endl;
}
curl_easy_cleanup(curl);
}
else {
std::cout << "Failed to initialize Curl." << std::endl;
return 1;
}
return 0;
}
```
在上述示例代码中,我们首先读取HAR文件并解析出URL。然后,使用Curl库初始化一个Curl实例,并设置URL、写入回调函数以及写入回调函数的数据。最后,使用`curl_easy_perform`函数发送HTTP请求并接收响应。你可以根据实际需求对代码进行修改和优化。
请注意,上述代码需要安装Curl库,并在编译时链接该库。你可以根据你的操作系统和开发环境选择合适的方式安装和使用Curl库。
阅读全文
相关推荐
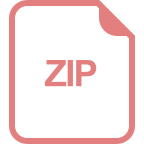
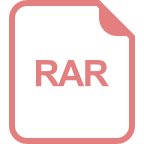
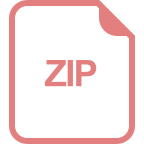
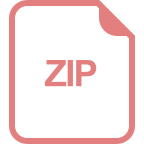
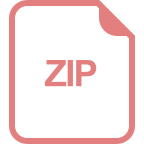
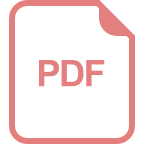
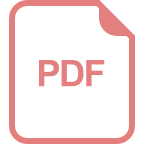
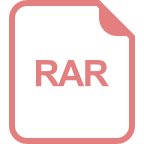
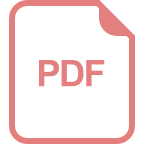
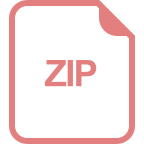
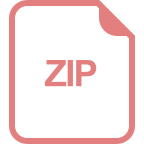
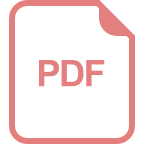