图灵机c++实现代码
时间: 2024-11-19 10:17:13 浏览: 35
图灵机是一种理论模型,用于描述计算过程,通常在计算机科学中作为计算复杂性的基础。要在C++中实现图灵机并不是直接的,因为它是理论模型,并不适合直接编写出实际运行的机器代码。然而,你可以创建一个模拟器或者抽象类来演示其工作原理。
下面是一个简单的概念性的C++代码片段,它展示了如何设计一个基本的图灵机抽象类:
```cpp
class TuringMachine {
public:
virtual ~TuringMachine() {}
// 状态转移函数,接受当前状态、输入字符和读写头位置,返回新的状态、写入的新字符和读写头移动的方向
virtual std::tuple<int, char, int> transition(int currentState, char inputChar, int tapeHeadPosition) = 0;
// 设置初始状态、输入字符串和读写头位置
void setInitialState(int initialState, const std::string& input, int tapeHeadPosition = 0) {
this->currentState = initialState;
this->tapeInput = input;
tapeHeadPosition_ = tapeHeadPosition;
}
// 模拟单一步骤的计算
void step() {
if (tapeInput.empty()) {
// 如果达到输入末尾,则处理特殊情况(例如halt)
processEndOfInput();
} else {
auto [newState, newChar, headMove] = transition(currentState, tapeInput[0], tapeHeadPosition_);
tapeInput.erase(0, 1);
tapeHeadPosition_ += headMove;
currentState = newState;
}
}
protected:
// 子类需要覆盖的处理输入结束的方法
virtual void processEndOfInput() = 0;
private:
int currentState;
std::string tapeInput;
int tapeHeadPosition_;
};
```
阅读全文
相关推荐
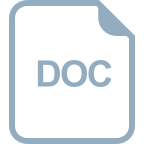
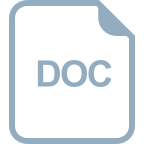
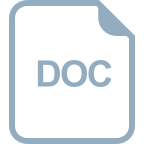
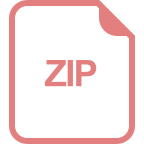

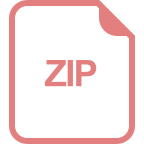
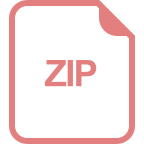
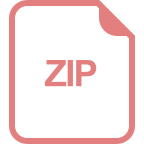
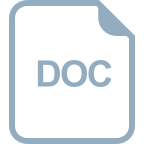
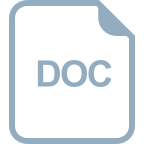
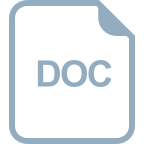
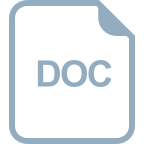
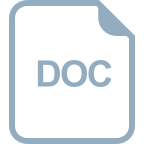
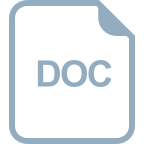
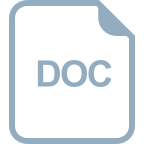
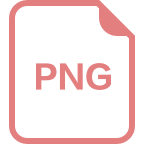