python俄罗斯方块毕设
时间: 2023-12-14 13:34:41 浏览: 111
以下是关于Python俄罗斯方块毕设的介绍:
Python俄罗斯方块毕设是一种基于Python语言编写的俄罗斯方块游戏,可以作为毕业设计项目来完成。该项目需要使用Pygame模块,这是一系列专门为编写电子游戏而设计的Python模块,它在已经非常优秀的SDL库的基础上增加了许多功能,让你能够用Python语言编写出丰富多彩的游戏程序。
在实现Python俄罗斯方块毕设时,你需要掌握以下技能:
1. Pygame模块的使用,包括游戏窗口的创建、游戏场景的绘制、游戏音效的播放等。
2. 俄罗斯方块游戏的规则和玩法,包括方块的移动、旋转、消除等。
3. Python语言的基础知识,包括变量、函数、条件语句、循环语句等。
以下是一个简单的Python俄罗斯方块游戏的代码示例,供参考:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
WINDOW_SIZE = (WINDOW_WIDTH, WINDOW_HEIGHT)
# 设置游戏场景大小
SCENE_WIDTH = 300
SCENE_HEIGHT = 600
SCENE_SIZE = (SCENE_WIDTH, SCENE_HEIGHT)
# 设置游戏窗口标题
pygame.display.set_caption('Python俄罗斯方块')
# 创建游戏窗口
screen = pygame.display.set_mode(WINDOW_SIZE)
# 加载游戏音效
pygame.mixer.music.load('bgm.mp3')
pygame.mixer.music.play(-1)
# 定义方块的形状
shapes = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]],
[[7, 7, 7, 7]]
]
# 定义方块的颜色
colors = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(128, 128, 128)
]
# 定义方块的大小
BLOCK_SIZE = 30
# 定义游戏场景
scene = [[0] * (SCENE_WIDTH // BLOCK_SIZE) for _ in range(SCENE_HEIGHT // BLOCK_SIZE)]
# 定义当前方块
current_shape = random.choice(shapes)
current_color = random.randint(1, len(colors) - 1)
current_x = SCENE_WIDTH // BLOCK_SIZE // 2 - len(current_shape[0]) // 2
current_y = 0
# 定义游戏循环
clock = pygame.time.Clock()
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 绘制游戏场景
screen.fill((255, 255, 255))
for y, row in enumerate(scene):
for x, block in enumerate(row):
if block > 0:
pygame.draw.rect(screen, colors[block], (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制当前方块
for y, row in enumerate(current_shape):
for x, block in enumerate(row):
if block > 0:
pygame.draw.rect(screen, colors[current_color], ((current_x + x) * BLOCK_SIZE, (current_y + y) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 更新游戏场景
if pygame.time.get_ticks() % 500 == 0:
if current_y + len(current_shape) < len(scene) and not any([scene[current_y + y][current_x + x] > 0 for y, row in enumerate(current_shape) for x, block in enumerate(row) if block > 0]):
current_y += 1
else:
for y, row in enumerate(current_shape):
for x, block in enumerate(row):
if block > 0:
scene[current_y + y][current_x + x] = current_color
current_shape = random.choice(shapes)
current_color = random.randint(1, len(colors) - 1)
current_x = SCENE_WIDTH // BLOCK_SIZE // 2 - len(current_shape[0]) // 2
current_y = 0
# 更新游戏窗口
pygame.display.update()
# 控制游戏帧率
clock.tick(60)
```
阅读全文
相关推荐
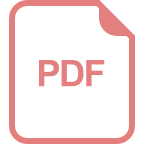

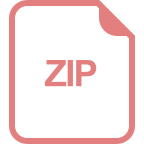
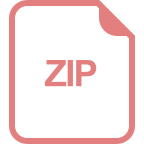
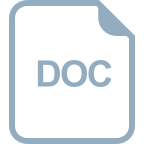
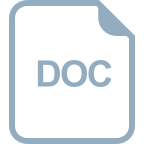
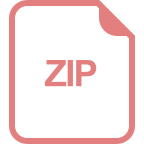
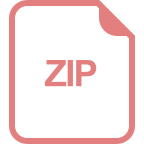
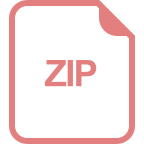
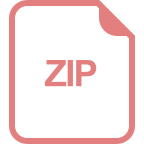
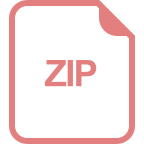
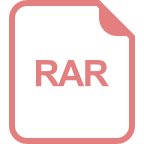
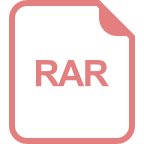
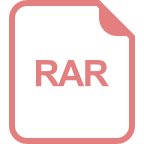
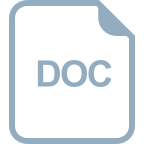
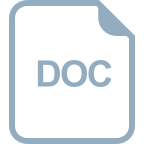