数据结构编写一个详细的c语言版通讯录管理系统
时间: 2025-01-05 13:44:05 浏览: 6
在C语言中,我们可以使用链表数据结构来编写一个简单的通讯录管理系统。这里是一个基本的设计概述:
首先,我们创建两个主要的数据结构:`Contact` 和 `AddressBook`。
```c
// Contact 结构体表示联系人,包含姓名和电话号码
typedef struct {
char name[50];
char phone_number[20];
} Contact;
// AddressBook 结构体代表通讯录,包含一个链表来存储联系人
typedef struct {
Contact* contacts;
int size;
int capacity; // 预留空间容量
} AddressBook;
```
接下来,我们提供一些基本操作函数:
1. **初始化** (`init_address_book()`):创建一个新的地址簿,并分配内存给联系人的链表。
```c
void init_address_book(AddressBook* book) {
book->contacts = NULL;
book->size = 0;
book->capacity = 10; // 初始容量
}
```
2. **添加联系人** (`add_contact()`):检查是否有足够的空间,如果没有,则扩大链表的容量。
```c
void add_contact(AddressBook* book, const char* name, const char* phone_number) {
if (book->size == book->capacity) {
resize_address_book(book);
}
Contact* new_contact = (Contact*)malloc(sizeof(Contact));
strcpy(new_contact->name, name);
strcpy(new_contact->phone_number, phone_number);
new_contact->next = book->contacts;
book->contacts = new_contact;
book->size++;
}
```
3. **查找联系人** (`find_contact()`):遍历链表查找指定名称的联系人。
```c
Contact* find_contact(AddressBook* book, const char* name) {
for (Contact* contact = book->contacts; contact != NULL; contact = contact->next) {
if (strcmp(contact->name, name) == 0) {
return contact;
}
}
return NULL;
}
```
4. **删除联系人** (`delete_contact()`):通过名字查找并从链表中移除联系人。
```c
void delete_contact(AddressBook* book, const char* name) {
for (Contact** current = &book->contacts; *current != NULL; ) {
Contact* temp = *current;
if (strcmp(temp->name, name) == 0) {
*current = temp->next;
free(temp); // 释放内存
book->size--;
} else {
current = &(*current)->next;
}
}
}
```
5. **显示所有联系人** (`display_contacts()`):遍历链表并打印每个联系人信息。
```c
void display_contacts(const AddressBook* book) {
Contact* contact = book->contacts;
while (contact != NULL) {
printf("%s - %s\n", contact->name, contact->phone_number);
contact = contact->next;
}
}
```
阅读全文
相关推荐




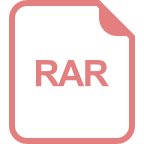











