用C语言写通讯录管理系统
时间: 2025-01-01 07:35:34 浏览: 24
在C语言中编写通讯录管理系统的基本步骤可以分为以下几个部分:
1. **数据结构设计**:首先,你可以创建一个结构体(struct),例如`Contact`,用于存储联系人的信息,如姓名、电话号码、电子邮件等。
```c
typedef struct {
char name[50];
char phone[20];
char email[50];
} Contact;
```
2. **初始化函数**:创建一个函数来添加新的联系人到列表中,如`add_contact`。
```c
void add_contact(Contact* list, int index, Contact new_contact) {
if (index < 0 || index > MAX_CONTACTS) {
printf("Invalid index.\n");
return;
}
list[index] = new_contact;
}
```
3. **查找功能**:提供函数来搜索特定的联系人,比如`search_contact`。
```c
Contact* search_contact(Contact* list, const char* name) {
for (int i = 0; i < MAX_CONTACTS; i++) {
if (!strcmp(list[i].name, name)) {
return &list[i];
}
}
return NULL;
}
```
4. **显示功能**:函数`display_contacts`用于遍历并打印联系人列表。
```c
void display_contacts(Contact* list) {
for (int i = 0; i < MAX_CONTACTS; i++) {
printf("%s - Phone: %s - Email: %s\n", list[i].name, list[i].phone, list[i].email);
}
}
```
5. **用户界面**:通过循环接收用户的输入,决定是添加、查找还是显示联系人,直到用户选择退出。
注意,这个例子是一个简化的版本,实际项目中还需要考虑错误处理、文件读写以持久化存储联系人等。此外,上述代码未包含动态内存分配,如果需要管理大型联系人列表,可能会使用链表或其他动态数据结构。
阅读全文
相关推荐








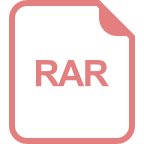







