帮我用C语言完成以下问题的代码:假设以块链结构(blocked linked)表示串,试编写将串 s 插入到串 t 中某个字符之后的算法,若该字符不存在,则将串 s 联接在串 t 之后。
时间: 2024-10-16 09:11:05 浏览: 55
在C语言中,我们可以使用链表和指针来实现这个功能。首先,我们需要定义一个链表节点结构,然后创建两个函数:一个用于插入字符串,另一个用于查找插入位置。如果插入位置的字符存在,就插入到其后面;否则,直接添加到串t的末尾。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
char data;
struct Node* next;
} BlockNode;
BlockNode* createNode(char c) {
BlockNode* newNode = (BlockNode*)malloc(sizeof(BlockNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = c;
newNode->next = NULL;
return newNode;
}
void insertString(BlockNode** head, char* strToInsert, BlockNode* target) {
if (!strToInsert || !target) {
return;
}
BlockNode* current = *head;
while (current != NULL && strcmp(current->data, strToInsert) <= 0) {
current = current->next;
}
if (current == NULL) { // 如果目标字符不存在,将字符串追加到末尾
BlockNode* newNode = createNode(*strToInsert);
if (newNode != NULL) {
if (*head == NULL) {
*head = newNode;
} else {
newNode->next = *head;
*head = newNode;
}
}
} else { // 将字符串插入到目标字符之后
BlockNode* newNode = createNode(*strToInsert);
if (newNode != NULL) {
newNode->next = current->next;
current->next = newNode;
}
}
}
// 示例用法
int main() {
char s[] = "insert";
char t[] = "test_string";
BlockNode* tHead = createNode(t[0]); // 创建链表头结点
for (size_t i = 1; t[i]; i++) {
tHead->next = createNode(t[i]);
tHead = tHead->next;
}
BlockNode* temp = tHead;
while (temp->next != NULL && temp->data != 's') { // 查找's'的位置
temp = temp->next;
}
insertString(&tHead, s, temp); // 插入's'
BlockNode* printList(BlockNode* head) {
while (head) {
printf("%c -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
printList(tHead); // 打印结果串t
return 0;
}
```
这个程序会将字符串`s`插入到串`t`中的第一个's'字符之后,如果't'中没有's',则`s`会被追加到`t`的末尾。
阅读全文
相关推荐
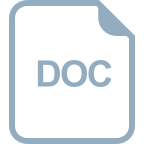
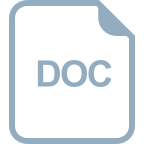
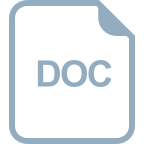
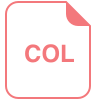
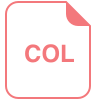
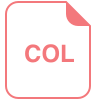
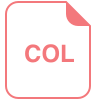

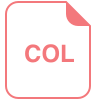
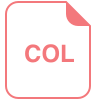
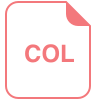







