帮用C语言我写一个点名的完整代码
时间: 2024-10-22 19:29:03 浏览: 12
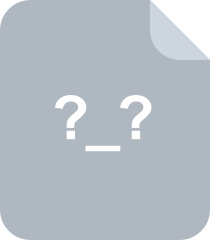
用C语言实现点名功能的完整代码

以下是两个部分的C语言点名器代码示例:
**部分一:基本结构与导入/导出名单**
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 定义学生结构体
typedef struct {
char name[50];
} Student;
// 读取文件到学生数组
void loadNames(char *filename, Student *students, int *numStudents) {
FILE *file = fopen(filename, "r");
if (file == NULL) {
printf("Error opening file.\n");
return;
}
*numStudents = fread(students, sizeof(Student), 1, file);
fclose(file);
}
// 写入学生数组到文件
void saveNames(char *filename, Student *students, int numStudents) {
FILE *file = fopen(filename, "w");
if (file == NULL) {
printf("Error creating file.\n");
return;
}
fwrite(students, sizeof(Student), numStudents, file);
fclose(file);
}
// 滚动点名
void rollCall(Student *students, int numStudents) {
// ...
}
// 随机点名
void randomRollCall(Student *students, int numStudents) {
srand(time(NULL));
int index = rand() % numStudents;
printf("Today's student is: %s\n", students[index].name);
}
```
**部分二:主函数实现**
```c
int main() {
const char* filename = "student_list.txt";
Student students[100]; // 假设最多有100个学生
int numStudents = 0;
// 加载学生名单
loadNames(filename, students, &numStudents);
// 开始点名循环
while (true) {
printf("\nChoose a mode: 1. Scroll 2. Random\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 1:
rollCall(students, numStudents);
break;
case 2:
randomRollCall(students, numStudents);
break;
default:
printf("Invalid choice.\n");
break;
}
// 询问是否保存点名结果
char saveChoice;
printf("Save current pointings? (y/n): ");
scanf(" %c", &saveChoice);
if (saveChoice == 'y') {
saveNames(filename, students, numStudents);
}
}
return 0;
}
```
阅读全文
相关推荐
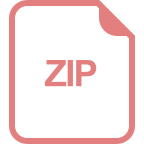
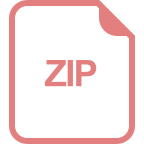

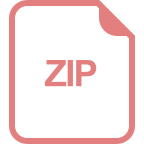
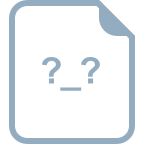
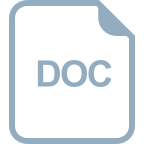
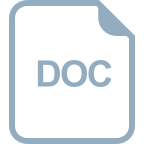
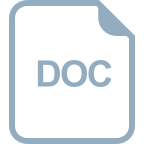








