用C语言实现凯撒密码算法,包括加密算法和解密算法。
时间: 2024-12-16 08:15:19 浏览: 14
凯撒密码是一种简单的替换式加密技术,通过将字母表中的每个字符按照固定位移进行替换来进行加密。以下是使用C语言实现的简单版本:
**加密算法(CaesarCipherEncrypt):**
```c
#include <stdio.h>
#include <string.h>
char shift(char c, int key) {
if (c >= 'A' && c <= 'Z') { // 大写字母
return ((c - 'A' + key) % 26) + 'A';
} else if (c >= 'a' && c <= 'z') { // 小写字母
return ((c - 'a' + key) % 26) + 'a';
}
return c; // 对于非字母字符不做处理
}
void caesar_encrypt(char* plaintext, int key, char* ciphertext) {
for (int i = 0; plaintext[i]; i++) {
ciphertext[i] = shift(plaintext[i], key);
}
ciphertext[i] = '\0'; // 添加终止符
}
int main() {
char plaintext[100];
printf("请输入明文: ");
fgets(plaintext, sizeof(plaintext), stdin); // 防止换行符
plaintext[strlen(plaintext) - 1] = '\0'; // 去掉换行符
int key;
printf("请输入偏移量: ");
scanf("%d", &key);
char ciphertext[100];
caesar_encrypt(plaintext, key, ciphertext);
printf("加密后的密文: %s\n", ciphertext);
return 0;
}
```
**解密算法(CaesarCipherDecrypt):**
```c
void caesar_decrypt(char* ciphertext, int key, char* plaintext) {
for (int i = 0; ciphertext[i]; i++) {
plaintext[i] = shift(ciphertext[i], -key); // 使用相反的偏移量
}
plaintext[i] = '\0';
}
int main() {
char ciphertext[100];
printf("请输入密文: ");
fgets(ciphertext, sizeof(ciphertext), stdin);
ciphertext[strlen(ciphertext) - 1] = '\0';
int key;
printf("请输入相同的偏移量用于解密: ");
scanf("%d", &key);
char plaintext[100];
caesar_decrypt(ciphertext, key, plaintext);
printf("解密后的明文: %s\n", plaintext);
return 0;
}
```
注意:以上代码仅适用于英文文本,对于其他语言或特殊字符的处理需要额外考虑。
阅读全文
相关推荐
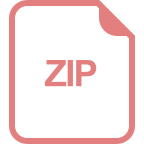
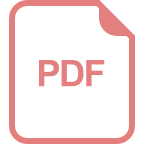
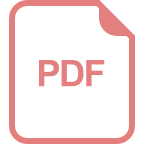
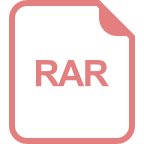
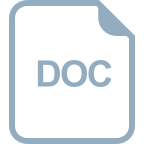
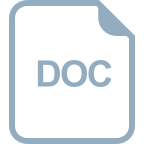



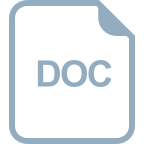
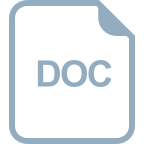
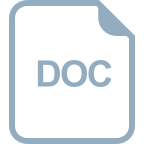
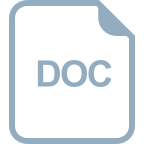
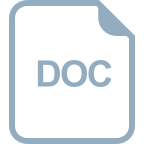
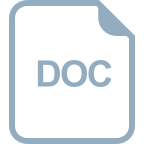

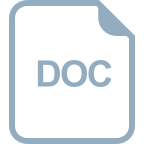
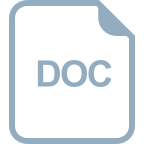