javafx大球吃小球代码
时间: 2024-07-16 12:00:34 浏览: 159
在JavaFX中实现"大球吃小球"的游戏通常会涉及到用户界面设计、事件处理和游戏逻辑等部分。以下是一个简化的代码示例,展示了如何创建基本的场景和控制球体的行为:
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
public class PacmanGame extends Application {
private static final int SIZE = 800;
private Circle pacman, ghost;
private double pacManSpeed = 2.0;
private double ghostSpeed = 1.0;
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Pacman Game");
primaryStage.setScene(createScene());
primaryStage.show();
}
private Scene createScene() {
Pane pane = new Pane();
pane.setWidth(SIZE);
pane.setHeight(SIZE);
pane.setStyle("-fx-background-color: #222222;");
pacman = new Circle(20, Color.WHITE, 10);
pacman.setTranslateX(Math.random() * SIZE);
pacman.setTranslateY(Math.random() * SIZE);
pane.getChildren().add(pacman);
// 假设有一个 Ghost 类来代表鬼魂,这里简化为一个圆形
ghost = new Circle(20, Color.RED, 10);
ghost.setTranslateX(Math.random() * (SIZE - 20));
ghost.setTranslateY(Math.random() * (SIZE - 20));
pane.getChildren().add(ghost);
pane.setOnMousePressed(e -> movePacman(e.getX(), e.getY()));
return new Scene(pane);
}
private void movePacman(double x, double y) {
double dx = x - pacman.getCenterX();
double dy = y - pacman.getCenterY();
if (Math.pow(dx, 2) + Math.pow(dy, 2) < 100) { // 当接近鬼魂时,移动速度减慢
pacManSpeed = 1.0;
} else {
pacManSpeed = 2.0;
}
pacman.setTranslateX(pacman.getTranslateX() + pacManSpeed * dx);
pacman.setTranslateY(pacman.getTranslateY() + pacManSpeed * dy);
}
public static void main(String[] args) {
launch(args);
}
}
```
阅读全文
相关推荐
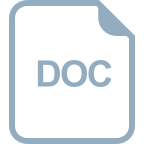
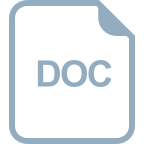
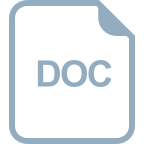

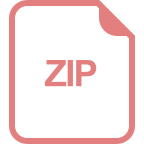
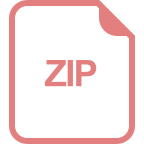
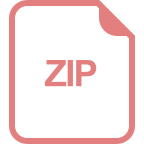
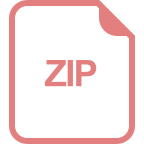
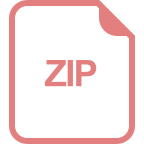
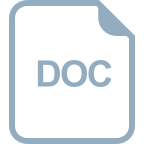
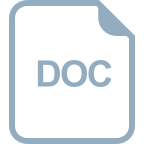
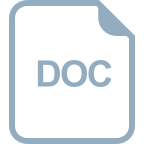
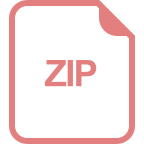
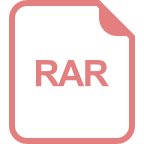
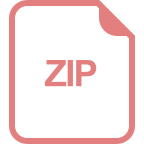
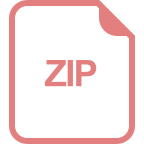
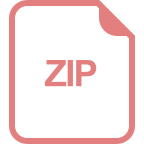
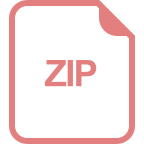