HAL_MODULE_INFO_SYM
时间: 2024-10-10 07:15:31 浏览: 37
HAL_MODULE_INFO_SYM是一个用于嵌入式系统软件框架,特别是像FreeRTOS、STM32Cube HAL等高级低级( HAL )库中的宏定义或结构体。它通常用来存储模块的相关信息,如函数指针、数据结构、配置选项等,以便于硬件抽象层(HAL)管理。这个宏或结构体包含了模块的基本描述,使得模块可以在整个软件堆栈中被识别和初始化。
举个例子,在STM32Cube HAL中,`HAL_MODULE_INFO_SYM`可能是这样一个结构:
```c
typedef struct {
/* 模块名称 */
const char* ModuleName;
/* 函数指针列表 */
void (*Init)(HAL_HandleTypeDef *hModule);
void (*DeInit)(HAL_HandleTypeDef *hModule);
/* 其他功能函数指针... */
} HAL_MODULE_INFOTypeDef;
```
通过这种方式,驱动程序开发者可以提供特定模块的初始化和卸载操作,而高层软件可以根据这个信息动态地调用相应的函数。
相关问题
audio HAL 2.0接口是怎样的
好的,以下是一个简单的 audio HAL 2.0 接口的示例:
```c++
#include <hardware/hardware.h>
#include <hardware/audio.h>
// 定义音频硬件抽象层(HAL)接口
struct audio_hw_device {
struct hw_device_t common;
// 添加音频通道(Audio Channel)接口
void (*register_audio_channel)(struct audio_hw_device* dev, audio_channel_t* channel);
void (*unregister_audio_channel)(struct audio_hw_device* dev, audio_channel_t* channel);
// 保留音频 HAL 1.0 接口
int (*set_voice_volume)(struct audio_hw_device* dev, float volume);
int (*set_master_volume)(struct audio_hw_device* dev, float volume);
int (*set_mode)(struct audio_hw_device* dev, audio_mode_t mode);
int (*set_mic_mute)(struct audio_hw_device* dev, bool state);
int (*set_parameters)(struct audio_hw_device* dev, const char* kvpairs);
char* (*get_parameters)(struct audio_hw_device* dev, const char* keys);
int (*set_input_device)(struct audio_hw_device* dev, audio_devices_t device);
int (*set_output_device)(struct audio_hw_device* dev, audio_devices_t device);
int (*init_check)(const struct audio_hw_device* dev);
};
// 定义音频通道(Audio Channel)接口
struct audio_channel {
audio_channel_handle_t handle;
void (*set_config)(struct audio_channel* channel, audio_config_t* config);
void (*get_config)(struct audio_channel* channel, audio_config_t* config);
};
// 定义音频硬件抽象层(HAL)模块
struct audio_module {
struct hw_module_t common;
};
// 定义音频硬件抽象层(HAL)模块方法
struct audio_module_methods {
int (*open)(const struct hw_module_t* module, const char* name, struct hw_device_t** device);
int (*close)(struct hw_device_t* device);
};
// 定义音频硬件抽象层(HAL)模块实例
struct audio_module HAL_MODULE_INFO_SYM = {
.common = {
.tag = HARDWARE_MODULE_TAG,
.module_api_version = AUDIO_MODULE_API_VERSION_2_0, // 升级到音频模块 API 版本 2.0
.hal_api_version = HARDWARE_HAL_API_VERSION,
.id = "audio.default",
.name = "Default audio HW HAL",
.author = "The Android Open Source Project",
.methods = &audio_module_methods,
},
};
// 定义音频硬件抽象层(HAL)模块方法实现
static struct audio_hw_device* open_audio_device(const struct hw_module_t* module, const char* name, uint32_t device) {
struct audio_hw_device* dev;
dev = (struct audio_hw_device*)calloc(1, sizeof(struct audio_hw_device));
dev->common.tag = HARDWARE_DEVICE_TAG;
dev->common.version = AUDIO_DEVICE_API_VERSION_2_0; // 升级到音频设备 API 版本 2.0
dev->common.module = (struct hw_module_t*)module;
dev->register_audio_channel = NULL;
dev->unregister_audio_channel = NULL;
dev->set_voice_volume = NULL;
dev->set_master_volume = NULL;
dev->set_mode = NULL;
dev->set_mic_mute = NULL;
dev->set_parameters = NULL;
dev->get_parameters = NULL;
dev->set_input_device = NULL;
dev->set_output_device = NULL;
dev->init_check = NULL;
return dev;
}
static int close_audio_device(struct audio_hw_device* dev) {
free(dev);
return 0;
}
static struct audio_module_methods audio_module_methods = {
.open = open_audio_device,
.close = close_audio_device,
};
```
这段代码演示了如何定义一个简单的 audio HAL 2.0 接口,它在 audio HAL 1.0 接口的基础上新增了音频通道(Audio Channel)接口。通过音频通道接口,音频 HAL 驱动程序可以管理多个音频通道,每个通道可以使用不同的音频格式和采样率。这样,音频 HAL 驱动程序可以更灵活地处理音频数据,提高音频性能。在实现音频 HAL 2.0 接口时,需要注意兼容性问题,以确保它与 Android 系统的其他组件正常运行。
书写hall传感器android hal层代码
以下是一个简单的Hall传感器在Android HAL层的代码示例:
```c
#include <hardware/hardware.h>
#include <hardware/sensors.h>
#include <fcntl.h>
#include <errno.h>
#include <math.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#define SENSOR_NAME "hall-sensor"
#define SENSOR_VENDOR "ACME"
#define SENSOR_VERSION 1
#define SENSOR_HANDLE 0
struct hall_sensor_context_t {
struct sensors_poll_device_t device;
sensors_event_t sensor_event;
int fd;
};
static int hall_sensor_open(const struct hw_module_t* module, const char* name,
struct hw_device_t** device);
static int hall_sensor_close(struct hw_device_t* device);
static int hall_sensor_activate(struct sensors_poll_device_t *dev,
int handle, int enabled);
static int hall_sensor_set_delay(struct sensors_poll_device_t *dev,
int handle, int64_t ns);
static int hall_sensor_poll(struct sensors_poll_device_t *dev,
sensors_event_t* data, int count);
static struct hw_module_methods_t hall_sensor_module_methods = {
.open = hall_sensor_open
};
struct sensors_poll_device_t HAL_MODULE_INFO_SYM = {
.common = {
.tag = HARDWARE_DEVICE_TAG,
.version = 0,
.module = &HAL_MODULE_INFO_SYM.common,
.close = hall_sensor_close,
},
.poll = hall_sensor_poll,
.activate = hall_sensor_activate,
.setDelay = hall_sensor_set_delay,
};
static int hall_sensor_open(const struct hw_module_t* module, const char* name,
struct hw_device_t** device) {
if (strcmp(name, SENSORS_POLL_DEVICE_NAME)) {
return -EINVAL;
}
struct hall_sensor_context_t* hall_dev = (struct hall_sensor_context_t*) malloc(sizeof(struct hall_sensor_context_t));
memset(hall_dev, 0, sizeof(*hall_dev));
hall_dev->device.common.tag = HARDWARE_DEVICE_TAG;
hall_dev->device.common.version = 0;
hall_dev->device.common.module = (struct hw_module_t*) module;
hall_dev->device.common.close = hall_sensor_close;
hall_dev->device.poll = hall_sensor_poll;
hall_dev->device.activate = hall_sensor_activate;
hall_dev->device.setDelay = hall_sensor_set_delay;
hall_dev->sensor_event.version = sizeof(sensors_event_t);
hall_dev->sensor_event.sensor = SENSOR_HANDLE;
hall_dev->sensor_event.type = SENSOR_TYPE_MAGNETIC_FIELD;
hall_dev->sensor_event.data[0] = 0.0f;
hall_dev->sensor_event.data[1] = 0.0f;
hall_dev->sensor_event.data[2] = 0.0f;
hall_dev->fd = open("/dev/hall-sensor", O_RDONLY);
if (hall_dev->fd < 0) {
ALOGE("Failed to open hall sensor device: %s", strerror(errno));
free(hall_dev);
return -errno;
}
*device = &hall_dev->device.common;
return 0;
}
static int hall_sensor_close(struct hw_device_t* device) {
struct hall_sensor_context_t* hall_dev = (struct hall_sensor_context_t*) device;
close(hall_dev->fd);
free(hall_dev);
return 0;
}
static int hall_sensor_activate(struct sensors_poll_device_t *dev,
int handle, int enabled) {
struct hall_sensor_context_t* hall_dev = (struct hall_sensor_context_t*) dev;
if (handle != SENSOR_HANDLE || (enabled != 0 && enabled != 1)) {
return -EINVAL;
}
return 0;
}
static int hall_sensor_set_delay(struct sensors_poll_device_t *dev,
int handle, int64_t ns) {
struct hall_sensor_context_t* hall_dev = (struct hall_sensor_context_t*) dev;
if (handle != SENSOR_HANDLE) {
return -EINVAL;
}
return 0;
}
static int hall_sensor_poll(struct sensors_poll_device_t *dev,
sensors_event_t* data, int count) {
struct hall_sensor_context_t* hall_dev = (struct hall_sensor_context_t*) dev;
ssize_t n = read(hall_dev->fd, &hall_dev->sensor_event.data[0], sizeof(float));
if (n < 0) {
ALOGE("Failed to read hall sensor data: %s", strerror(errno));
return -errno;
}
hall_dev->sensor_event.timestamp = getTimestamp();
*data = hall_dev->sensor_event;
return 1;
}
```
这个代码示例定义了一个名为`hall-sensor`的传感器,它返回磁场强度值。在`hall_sensor_open()`函数中,我们打开了`/dev/hall-sensor`设备文件,并初始化了一个`sensors_event_t`结构体来存储传感器事件。在`sensors_poll_device_t`结构体中,我们定义了传感器的`poll()`、`activate()`和`setDelay()`函数。在`hall_sensor_activate()`和`hall_sensor_set_delay()`函数中,我们简单地检查传入的`handle`参数是否是我们定义的传感器句柄,并返回0或-EINVAL。在`hall_sensor_poll()`函数中,我们从设备文件中读取传感器数据,并将其存储在先前初始化的`sensors_event_t`结构体中,最后返回1表示有一个新的传感器事件。
阅读全文
相关推荐
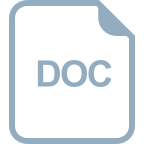
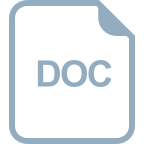
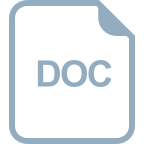
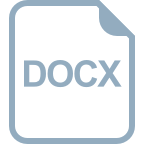
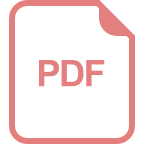
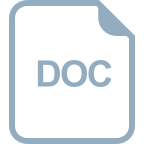



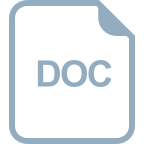
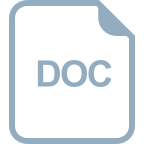
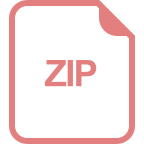
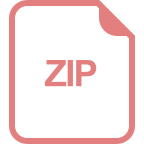
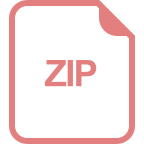