在python中怎么用dlib库实现对视频人脸的检测
时间: 2024-10-08 14:07:10 浏览: 32
在Python中使用dlib库实现视频中的人脸检测,你需要先安装dlib库和其附带的脸部关键点检测模型。以下是简单的步骤:
1. **安装必要的库**:
- 首先,确保已经安装了`pip`。如果没有,可以通过Python的包管理器安装。然后,安装dlib和它的 frontalface detector模型:
```
pip install dlib numpy opencv-python-headless
```
2. **下载预训练模型**:
- dlib官方提供了一个预训练的人脸检测模型,名为`sphereface模型`。这个模型可以在dlib GitHub仓库找到:https://github.com/davisking/dlib
3. **导入所需模块并加载模型**:
```python
import cv2
import dlib
from imutils import face_utils
# 加载面部检测器
predictor_path = "shape_predictor_68_face_landmarks.dat" # 使用预训练的面部特征预测模型
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor(predictor_path)
# 初始化摄像头或读取视频文件
cap = cv2.VideoCapture('video.mp4')
```
4. **实时人脸检测**:
```python
while True:
ret, frame = cap.read()
if not ret:
break
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 进行人脸检测
faces = detector(gray)
for face in faces:
# 获取面部的关键点并画出矩形框
landmarks = predictor(gray, face)
landmarks = face_utils.shape_to_np(landmarks)
cv2.rectangle(frame, (face.left(), face.top()), (face.right(), face.bottom()), (0, 255, 0), 2)
# 可选地,你可以在此处处理关键点数据或显示它们
cv2.imshow("Face Detection", frame)
key = cv2.waitKey(1) & 0xFF
if key == ord('q'):
break
```
5. **结束循环并释放资源**:
```
cap.release()
cv2.destroyAllWindows()
```
注意:以上代码假设视频文件存在且能正常播放。如果你需要从摄像头捕获视频,只需将`cap = cv2.VideoCapture('video.mp4')`替换为`cap = cv2.VideoCapture(0)`,其中0表示内置摄像头。
阅读全文
相关推荐
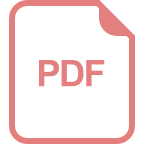
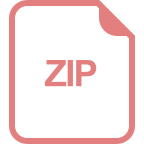
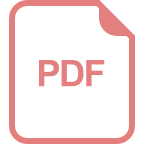
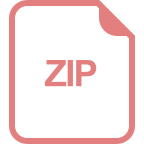
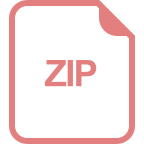
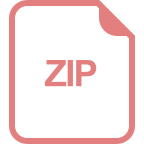
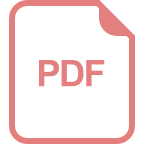
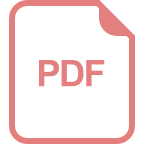
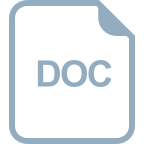
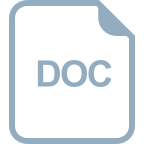
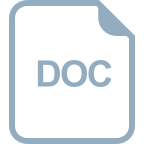
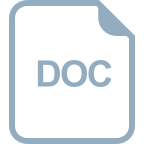

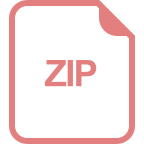

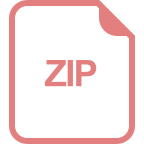
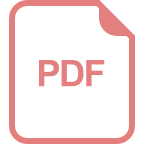
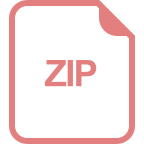