Spring Boot设计Comment类,包含评论的文本、作者、评论时间等属性。实现POST /api/statuses端点,创建新动态。
时间: 2024-09-12 16:07:48 浏览: 33
在Spring Boot中设计Comment类,首先需要定义该类的属性,比如评论文本(text)、作者(user)、评论时间(time)等。同时,可能还需要关联到某个特定的动态(status)。下面是一个简单的Comment类的示例:
```java
import javax.persistence.*;
import java.util.Date;
@Entity
public class Comment {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String text; // 评论文本
@ManyToOne(optional = false)
@JoinColumn(name = "user_id")
private User user; // 评论的作者
@Column(nullable = false)
@Temporal(TemporalType.TIMESTAMP)
private Date time; // 评论时间
// 这里可能还会有与Status实体的关联,省略...
// 省略构造方法、getter和setter方法
}
```
实现`/api/statuses`端点的POST方法,用于创建新的动态评论,可以在对应的Controller中添加一个处理POST请求的方法。这个方法接收Comment对象作为参数,并使用Service层处理逻辑。以下是一个简单的Controller方法的示例:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/statuses")
public class StatusController {
private final CommentService commentService;
@Autowired
public StatusController(CommentService commentService) {
this.commentService = commentService;
}
@PostMapping("/{statusId}/comments")
public Comment createComment(@PathVariable Long statusId, @RequestBody Comment comment) {
// 在实际应用中,这里可能需要处理关联到动态的逻辑,以及其他必要的验证
return commentService.saveComment(statusId, comment);
}
}
```
然后需要实现Service层的`saveComment`方法,该方法负责将传入的Comment对象保存到数据库中:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class CommentService {
private final CommentRepository commentRepository;
@Autowired
public CommentService(CommentRepository commentRepository) {
this.commentRepository = commentRepository;
}
public Comment saveComment(Long statusId, Comment comment) {
comment.setStatusId(statusId);
return commentRepository.save(comment);
}
}
```
注意:上面的代码只是一个简单的示例,实际应用中可能需要处理更多的细节,例如权限验证、异常处理、数据验证等。
阅读全文
相关推荐
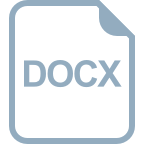
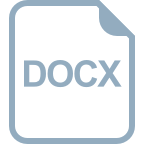
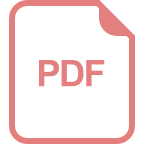
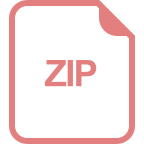
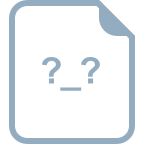
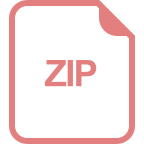
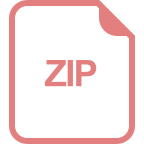
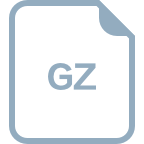
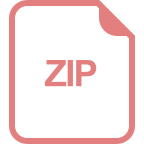
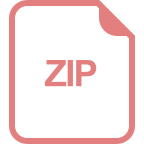
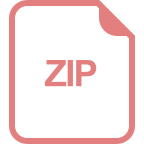
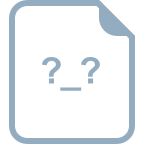
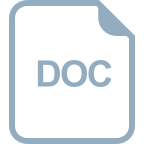
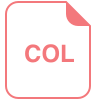




