低通滤波器OpenCVc++
时间: 2023-09-20 10:14:17 浏览: 59
低通滤波器是一种常用的图像处理技术,它可以平滑图像并降低图像中高频噪声的影响。在OpenCV中,你可以使用C++编写代码来实现低通滤波器。
首先,你需要包含OpenCV库的头文件:
```cpp
#include <opencv2/opencv.hpp>
```
然后,你可以使用`blur`函数来实现简单的均值滤波器。以下是一个示例代码:
```cpp
cv::Mat image = cv::imread("input.jpg");
cv::Mat blurredImage;
cv::blur(image, blurredImage, cv::Size(5, 5)); // 使用5x5的内核进行均值滤波
cv::imshow("Blurred Image", blurredImage);
cv::waitKey(0);
```
在上面的代码中,我们首先读取了一张图像,然后使用`blur`函数对图像进行了均值滤波。第三个参数表示内核的大小,这里我们使用了一个5x5的内核。最后,我们展示了滤波后的图像并等待用户按下任意键关闭窗口。
除了均值滤波器之外,OpenCV还提供了其他类型的低通滤波器,如高斯滤波器和中值滤波器。你可以根据自己的需求选择适合的滤波器。
希望这个回答能够帮到你!如果有任何问题,请随时提问。
相关问题
opencv巴特沃兹低通滤波器python代码
以下是使用OpenCV实现巴特沃兹低通滤波器的Python代码:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.jpg', 0)
# 定义巴特沃兹低通滤波器
def butterworth_lowpass_filter(img, d, n):
rows, cols = img.shape
crow, ccol = rows // 2, cols // 2
# 构建巴特沃兹低通滤波器
u, v = np.meshgrid(np.arange(cols), np.arange(rows))
d_uv = np.sqrt((u - ccol) ** 2 + (v - crow) ** 2)
H = 1 / (1 + (d / d_uv) ** (2 * n))
# 将滤波器应用于图像
img_fft = np.fft.fft2(img)
img_fft_shift = np.fft.fftshift(img_fft)
img_fft_shift_filtered = img_fft_shift * H
img_fft_filtered = np.fft.ifftshift(img_fft_shift_filtered)
img_filtered = np.fft.ifft2(img_fft_filtered)
img_filtered = np.abs(img_filtered)
return img_filtered
# 调用巴特沃兹低通滤波器函数
img_filtered = butterworth_lowpass_filter(img, 50, 2)
# 显示原始图像和滤波后的图像
cv2.imshow('Original Image', img)
cv2.imshow('Butterworth Lowpass Filtered Image', img_filtered)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`butterworth_lowpass_filter`函数接受三个参数:输入图像`img`、截止频率`d`和阶数`n`。函数返回经过巴特沃兹低通滤波器处理后的图像。
用opencv对图像进行butterworth低通滤波器图像平滑代码
下面是使用 OpenCV 实现 Butterworth 低通滤波器图像平滑的代码:
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg', 0) # 读取灰度图像
rows, cols = img.shape # 获取图像的行数和列数
crow, ccol = rows//2, cols//2 # 计算图像中心点坐标
# 构建 Butterworth 低通滤波器
d0 = 50 # 截止频率
n = 2 # 阶数
butterworth_lowpass = np.zeros((rows, cols, 2), np.float32)
for i in range(rows):
for j in range(cols):
distance = np.sqrt((i-crow)**2 + (j-ccol)**2)
butterworth_lowpass[i,j,0] = 1 / (1 + (distance/d0)**(2*n))
butterworth_lowpass[i,j,1] = 1 / (1 + (distance/d0)**(2*n))
# 将图像转换为频域
img_fft = np.fft.fft2(img)
# 将低通滤波器与图像频域相乘
img_fft_shift = np.fft.fftshift(img_fft)
img_filtered = img_fft_shift * butterworth_lowpass
# 将滤波后的图像转换回空域
img_back = np.fft.ifft2(np.fft.ifftshift(img_filtered))
img_filtered = np.real(img_back) # 取实部
# 显示原始图像和滤波后的图像
cv2.imshow('Original Image', img)
cv2.imshow('Filtered Image', img_filtered.astype(np.uint8))
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`d0` 为截止频率,`n` 为阶数,可以根据需要进行调整。滤波器的形状由截止频率和阶数共同决定,当 `n=1` 时,Butterworth 滤波器与高斯滤波器具有相似的形状。
相关推荐
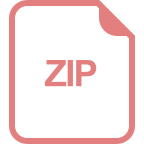
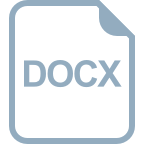












