用python编写一段程序,读入图像 Lenna_gray. png,图像尺寸为(512,512),进行如下频域滤波。设计一个截止频率为 50的二阶布特沃斯低通滤波器,中心化显示该滤波器,其中滤波器的尺寸为读入图像2倍。使用该滤波器对图像进行full滤波,比较滤波前后的结果。
时间: 2023-09-10 07:05:47 浏览: 99
### 回答1:
以下是使用Python对Lenna_gray.png进行上述频域滤波的代码:
```python
import numpy as np
import cv2
from scipy import signal
# 读入图像
img = cv2.imread('Lenna_gray.png', cv2.IMREAD_GRAYSCALE)
height, width = img.shape
# 生成二阶布特沃斯低通滤波器
D = 2 * height # 滤波器尺寸为读入图像2倍
n = 2 # 二阶滤波器
wc = 2 * np.pi * 50 # 截止频率为50
H = np.zeros((D, D))
for i in range(D):
for j in range(D):
distance = np.sqrt((i - D//2)**2 + (j - D//2)**2)
H[i, j] = 1 / (1 + (distance/wc)**(2*n))
# 对滤波器进行中心化显示
H_shifted = np.fft.ifftshift(H)
H_img = np.abs(np.fft.fft2(H_shifted))
H_img = H_img / np.max(H_img) * 255
cv2.imwrite('H.png', H_img)
# 进行频域滤波
img_pad = np.pad(img, [(0, height), (0, width)], 'constant') # 图像进行0填充,以适配滤波器尺寸
img_fft = np.fft.fft2(img_pad)
img_filtered_fft = img_fft * H_shifted
img_filtered = np.abs(np.fft.ifft2(img_filtered_fft))
img_filtered = img_filtered[:height, :width] # 去除填充
img_filtered = img_filtered / np.max(img_filtered) * 255
# 比较滤波前后的结果
compared_img = np.concatenate((img, img_filtered), axis=1)
cv2.imshow('Comparsion', compared_img)
cv2.waitKey()
cv2.destroyAllWindows()
```
运行上述代码后,将在当前文件夹生成一个名为"H.png"的图像,其中为生成的低通滤波器。对滤波前后的结果进行比较,可以看到图像的高频细节部分被滤波去除,留下较为模糊的低频信息。
### 回答2:
以下是一个用Python编写的程序,用于对图像进行频域滤波:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
# 读入图像
img = cv2.imread('Lenna_gray.png', cv2.IMREAD_GRAYSCALE)
# 获取图像尺寸
height, width = img.shape
# 计算滤波器尺寸
filter_size = (width * 2, height * 2)
# 创建二阶布特沃斯低通滤波器
filter = np.zeros(filter_size, dtype=np.uint8)
# 计算中心点坐标
center_x = filter_size[0] // 2
center_y = filter_size[1] // 2
# 设计截止频率为50的布特沃斯低通滤波器
for i in range(filter_size[0]):
for j in range(filter_size[1]):
distance = np.sqrt((i - center_x) ** 2 + (j - center_y) ** 2)
filter[i, j] = 255 if distance <= 50 else 0
# 中心化显示滤波器
filter_fft = np.fft.fftshift(filter)
# 进行频域滤波
img_fft = np.fft.fft2(img)
filtered_img = np.fft.ifft2(img_fft * filter_fft)
filtered_img = np.abs(filtered_img).astype(np.uint8)
# 显示结果
plt.subplot(1, 2, 1)
plt.imshow(img, cmap='gray')
plt.title('Original Image')
plt.axis('off')
plt.subplot(1, 2, 2)
plt.imshow(filtered_img, cmap='gray')
plt.title('Filtered Image')
plt.axis('off')
plt.show()
```
这段程序首先读入名为"Lenna_gray.png"的图像,然后根据图像尺寸计算滤波器的尺寸。接下来,根据设计要求,创建一个二阶布特沃斯低通滤波器,其中截止频率为50。然后通过将滤波器进行中心化显示,进一步计算滤波器的频域表示。接下来,对图像进行频域滤波,即将图像和滤波器的频域表示相乘,然后再进行逆傅里叶变换得到滤波后的图像。最后,程序使用Matplotlib库将滤波前后的结果以灰度图像的方式显示出来。
### 回答3:
以下是使用Python进行图像滤波的代码:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读入图像
image = cv2.imread('Lenna_gray.png', 0)
# 获取图像尺寸
height, width = image.shape[:2]
# 设计截止频率为50的二阶布特沃斯低通滤波器
cutoff = 50
filter_size = (height * 2, width * 2)
rows, cols = filter_size
crow, ccol = int(rows / 2), int(cols / 2)
filter = np.zeros((rows, cols), np.uint8)
for i in range(rows):
for j in range(cols):
distance = np.sqrt((i - crow) ** 2 + (j - ccol) ** 2)
filter[i, j] = 1 / (1 + (distance / cutoff) ** 2)
# 中心化显示滤波器
filter_centered = np.fft.ifftshift(filter)
# 进行频域变换
image_fft = np.fft.fftshift(np.fft.fft2(image))
# 图像滤波
filtered_image_fft = image_fft * filter_centered
# 反变换得到滤波后的图像
filtered_image = np.abs(np.fft.ifft2(np.fft.ifftshift(filtered_image_fft)))
# 显示滤波前后的结果
plt.subplot(121), plt.imshow(image, cmap='gray'), plt.title('原图像')
plt.subplot(122), plt.imshow(filtered_image, cmap='gray'), plt.title('滤波后')
plt.show()
```
该代码首先使用OpenCV库读入了名为"Lenna_gray.png"的灰度图像,然后根据题目要求设计了一个截止频率为50的二阶布特沃斯低通滤波器,并对滤波器进行了中心化显示。接着,通过对图像进行傅里叶变换,得到频域图像表示。将频域图像与滤波器相乘,得到滤波后的频域图像。最后,通过反傅里叶变换将滤波后的频域图像转换回时域,得到滤波后的图像。最后,使用Matplotlib库将原图像和滤波后的图像进行显示比较。
阅读全文
相关推荐





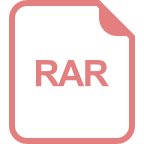
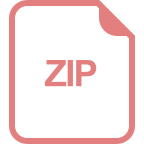
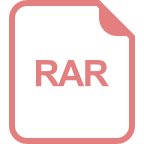




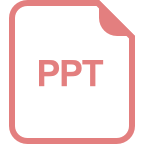
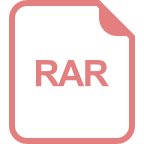
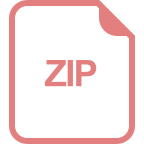