不用string函数库也不自定义函数 输入一个字符串,统计其中有多少个单词。单词之 间用空格分隔开。
时间: 2024-11-22 21:34:18 浏览: 20
为了统计一个字符串中的单词数,你可以采用逐字符遍历的方式,遇到空格就认为是一个单词的结束,然后开始计数新的单词。以下是Python的一个简单示例:
```python
def count_words(s):
word_count = 0
if s and s[0] != ' ': # 如果字符串非空且第一个字符不是空格,则开始计数
word_count += 1
for char in s[1:]:
if char == ' ': # 遇到空格则增加单词数
word_count += 1
# 最后检查一下字符串末尾是否还有单词
if s[-1] != ' ':
word_count += 1
return word_count
# 使用示例
input_string = "这是一个测试字符串"
word_count = count_words(input_string)
print("输入字符串中有", word_count, "个单词。")
```
这个函数会忽略字符串开头和结尾的空格,并只计算实际单词的数量。
相关问题
c++输入一行字符,统计其中有多少个单词,每两个单词之间以空格隔开。
可以使用C++的string和stringstream库来实现输入一行字符并统计单词个数的功能。具体代码如下:
```cpp
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int main() {
string line;
getline(cin, line); // 输入一行字符
stringstream ss(line); // 将字符串转换为stringstream对象
int wordCount = 0;
string word;
while (ss >> word) { // 每次读取一个单词
wordCount++;
}
cout << "单词个数为:" << wordCount << endl;
return 0;
}
```
以上代码中,我们首先使用getline函数读取一行字符,然后使用stringstream库将字符串转换为stringstream对象,方便我们按照空格分割字符串并统计单词个数。最后输出单词个数即可。
c++输入一行字符,计算该行字符中包含多少个单词,单词之间用空格分隔开。
在 C++ 中,你可以通过标准库中的 `cin` 和 `getline` 函数读取一行用户输入,并使用字符串流(如 `istringstream`)将这一行输入分割成单词。以下是实现的一个简单示例:
```cpp
#include <iostream>
#include <sstream>
#include <string>
int count_words(const std::string& input) {
int word_count = 0;
std::istringstream iss(input);
std::string word;
// 使用 iss 逐个读取单词直到遇到非单词字符(即不是字母、数字或下划线)
while (iss >> word) {
++word_count; // 每找到一个单词就增加计数器
}
return word_count;
}
int main() {
std::string line;
std::cout << "请输入一行字符(单词由空格分隔):" << std::endl;
getline(std::cin, line);
int word_count = count_words(line);
std::cout << "该行包含的单词数为: " << word_count << std::endl;
return 0;
}
```
在这个程序中,`count_words` 函数会统计输入字符串中有效单词的数量。注意,这个函数假设单词是由字母、数字或下划线组成的,且单词间用空格分隔。
阅读全文
相关推荐













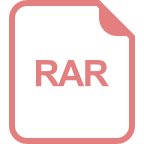


