arr[i] = arr[i] ^ arr[j]; arr[j] = arr[i] ^ arr[j]; arr[i] = arr[i] ^ arr[j];
时间: 2024-08-14 07:07:49 浏览: 31
这段代码是用于实现异或(XOR)操作的一种加密或混淆数组元素的方法,通常在一些简单的密码学算法中看到,如凯撒密码或简单的位操作。下面是这个过程的描述:
假设我们有一个数组`arr`,`i`和`j`是数组的索引。这里做的是一个循环,每次更新数组中索引为`i`和`j`的元素:
1. `arr[i] = arr[i] ^ arr[j];`[^4]
这一行将索引`i`处的元素与索引`j`处的元素执行异或运算,并将结果存储回索引`i`处。这样,原来`i`位置的元素就被改变了。
2. `arr[j] = arr[i] ^ arr[j];`[^4]
接下来,将之前`i`处的新值(经过异或后的结果)再次与原始的`j`处的元素执行异或运算,再把结果写回`j`位置。这一步又改变了`j`位置的元素。
3. `arr[i] = arr[i] ^ arr[j];`[^4]
最后一次,`i`处的元素会被它自己和`j`处当前值再次异或,完成一个完整的循环操作。
这种操作会使得`arr[i]`和`arr[j]`之间的关系变得复杂,但不会改变其他未参与异或的元素。如果要恢复原始值,通常需要知道初始状态或者额外的解密信息。
相关问题
调用这个sway方法实现数据交换,public static void sway(int[] arr,int i,int j){ if(i!=j){ //不能两个值指向同一地址 arr[i]=arr[i]^arr[j]; arr[j]=arr[i]^arr[j];//就是arr[i]^arr[j]^arr[j]就表示a arr[i]=arr[i]^arr[j];//表示arr[i]^arr[j]^arr[i]^arr[j]^arr[j]就是b } }
这个方法可以用来将数组中的两个元素进行交换。具体实现是利用异或运算,将两个元素的值进行异或操作,得到的结果再赋值回去。代码如下:
public static void sway(int[] arr, int i, int j){
if(i != j){
arr[i] = arr[i] ^ arr[j];
arr[j] = arr[i] ^ arr[j];
arr[i] = arr[i] ^ arr[j];
}
}
其中,^ 表示异或运算,其逻辑如下:
0 ^ 0 = 0
0 ^ 1 = 1
1 ^ 0 = 1
1 ^ 1 = 0
利用这个逻辑,我们可以实现两个值的交换,而不需要引入额外的变量。举个例子,如果我们要将 a 和 b 两个变量的值进行交换,代码如下:
a = a ^ b; // a = a^b
b = a ^ b; // b = a^b^b = a
a = a ^ b; // a = a^b^a = b
这样,变量 a 和 b 的值就完成了交换。同样的,我们可以利用这个方法实现数组元素的交换。
public static void sway(int[] arr,int i,int j){ if(i!=j){ //不能两个值指向同一地址 arr[i]=arr[i]^arr[j]; arr[j]=arr[i]^arr[j];//就是arr[i]^arr[j]^arr[j]就表示a arr[i]=arr[i]^arr[j];//表示arr[i]^arr[j]^arr[i]^arr[j]^arr[j]就是b } }
This is a Java method that takes an integer array, and two indices i and j as input parameters. The method swaps the values at the i-th and j-th indices of the array using bitwise XOR operations.
The method first checks if i and j are not equal, as swapping two values at the same index would result in both values being set to the same value. If i and j are different, the method performs the following operations:
1. The value at index i is XORed with the value at index j, and the result is stored in the value at index i.
2. The value at index j is XORed with the new value at index i, and the result is stored in the value at index j.
3. The value at index i is XORed with the new value at index j, and the result is stored in the value at index i.
These three operations effectively swap the values at indices i and j without using a temporary variable.
阅读全文
相关推荐
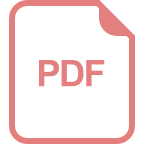
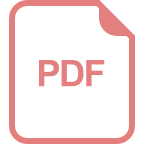
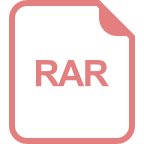














