c++使用opencv在图上标数字
时间: 2024-11-27 20:11:43 浏览: 0
在C++中使用OpenCV(Open Source Computer Vision Library)在图像上添加数字通常涉及到以下几个步骤:
1. **引入库**:
首先,需要包含必要的OpenCV头文件以及iostream用于输入输出。
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
```
2. **读取图像**:
使用`cv::imread()`函数加载图像。
```cpp
cv::Mat img = cv::imread("path_to_your_image.jpg");
if (img.empty()) {
std::cerr << "Could not read the image" << std::endl;
return -1;
}
```
3. **设置字体和颜色**:
`cv::FONT_HERSHEY_SIMPLEX`是常用的字体风格,可以设置字体大小、粗细和颜色。
```cpp
cv::Point pos(50, 50); // 起始位置
cv::Scalar color(0, 255, 0); // 绿色文字颜色
int fontScale = 1; // 字体大小
int thickness = 2; // 字体线宽
cv::Scalar fontColor(color); // 文字颜色
cv::Font font(cv::FONT_HERSHEY_SIMPLEX, fontScale, thickness, fontColor);
```
4. **在图像上绘制文本**:
使用`putText()`函数将数字标注在图像上。
```cpp
std::string strNum = "123"; // 想要在图像上显示的数字
cv::putText(img, strNum, pos, font, 1.0);
```
5. **保存结果**:
画好标签后的图像可以保存到新的文件或者直接显示出来。
```cpp
cv::imwrite("output_with_number.png", img);
// 或者
cv::imshow("Image with Number", img);
cv::waitKey(0); // 显示窗口等待按键关闭
```
完整示例代码可能如下所示:
```cpp
int main() {
// ... (步骤2-5)
cv::putText(img, "5", cv::Point(img.cols / 2, img.rows * 0.8), font, 1.0);
// ... (步骤5)
return 0;
}
```
阅读全文
相关推荐
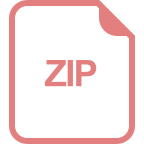
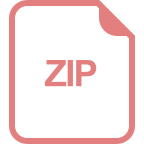
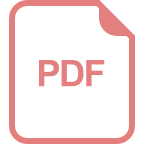
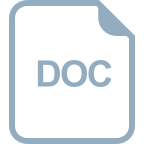
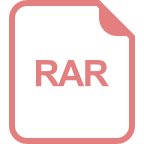
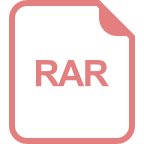
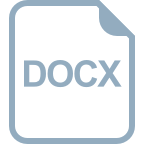
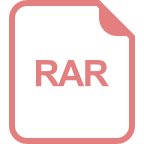
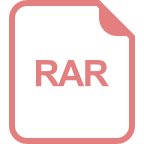
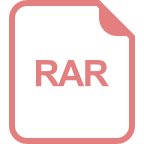
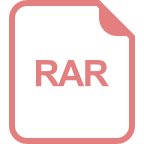
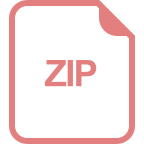
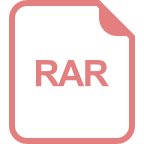
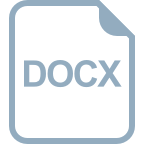
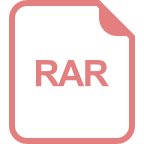