python DAG
时间: 2023-11-13 09:58:19 浏览: 144
Python DAG是用Python编程语言创建和处理DAG(有向无环图)的框架。它可以用于设计复杂而又可扩展的任务处理系统,并与丰富的库、API和插件生态系统一起使用。Python DAG提供了一种处理单个任务依赖的复杂处理流程的方式。它是用Python编写的,因此具有Python的灵活性和易于学习和使用的优势。学习Python DAG的步骤包括了解DAG的基本概念、学习Python编程语言、了解DAG开发的库和工具以及查看示例代码。尝试使用Python DAG开发自己的任务处理系统,可以帮助巩固所学知识。
相关问题
python DAG 最短路径
在有向无环图(DAG)中,最短路径问题是指从起点到终点的最短路径问题。DAG最短路径算法可以通过拓扑排序来实现。具体来说,我们可以先对DAG进行拓扑排序,然后按照拓扑排序的顺序依次更新每个节点的最短路径。在更新每个节点的最短路径时,我们只需要考虑该节点的所有入边即可。这个算法的时间复杂度为O(V+E),其中V是节点数,E是边数。
下面是Python实现DAG最短路径算法的代码:
```
from collections import defaultdict
def dag_shortest_path(graph, start):
# 拓扑排序
def topological_sort(graph):
in_degree = defaultdict(int)
for u in graph:
for v in graph[u]:
in_degree[v] += 1
queue = [u for u in graph if in_degree[u] == 0]
result = []
while queue:
u = queue.pop(0)
result.append(u)
for v in graph[u]:
in_degree[v] -= 1
if in_degree[v] == 0:
queue.append(v)
return result
# 初始化距离为无穷大
dist = {u: float('inf') for u in graph}
dist[start] = 0
# 按照拓扑排序的顺序更新距离
for u in topological_sort(graph):
for v in graph[u]:
dist[v] = min(dist[v], dist[u] + graph[u][v])
return dist
```
DAG python
In Python, a DAG (Directed Acyclic Graph) can be represented using various data structures or libraries. One popular library for working with DAGs is NetworkX.
Here's an example of how you can create a simple DAG using NetworkX in Python:
```python
import networkx as nx
# Create a directed acyclic graph
dag = nx.DiGraph()
# Add nodes to the graph
dag.add_nodes_from([1, 2, 3, 4])
# Add edges to represent dependencies
dag.add_edges_from([(1, 2), (1, 3), (2, 4), (3, 4)])
# Check if the graph is acyclic
is_acyclic = nx.is_directed_acyclic_graph(dag)
print("Is the graph acyclic?", is_acyclic)
# Perform other operations on the DAG
# ...
```
In this example, we create a directed acyclic graph and add nodes (1, 2, 3, and 4) to it. We then add edges to represent dependencies between the nodes. Finally, we check if the graph is acyclic using the `is_directed_acyclic_graph()` function provided by NetworkX.
You can perform various operations on the DAG using NetworkX, such as topological sorting, finding shortest paths, or visualizing the graph.
I hope this helps! Let me know if you have any further questions.
阅读全文
相关推荐
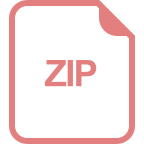
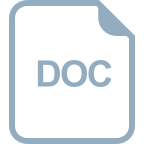
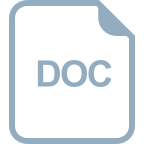
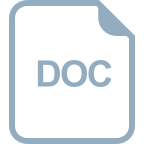





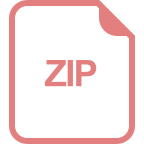
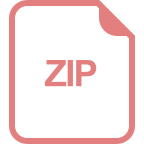
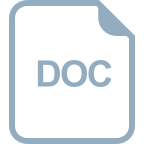
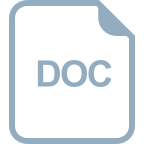
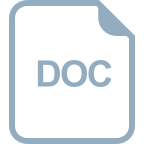
