c++string类型大小写转换
时间: 2023-11-18 17:52:53 浏览: 55
可以使用C++标准库中的`std::transform`函数来实现字符串大小写转换。具体实现如下:
```c++
#include <algorithm>
#include <string>
std::string str = "Hello World!";
std::transform(str.begin(), str.end(), str.begin(), ::tolower); // 转换为小写
std::transform(str.begin(), str.end(), str.begin(), ::toupper); // 转换为大写
```
其中,`::tolower`和`::toupper`是C++标准库中的函数,用于将字符转换为小写或大写。
相关问题
c++ string 大小写转换
你可以使用C++的标准库中的函数来进行字符串大小写转换。下面是一些示例代码:
1. 转换为大写字母:
```cpp
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string str = "Hello World!";
for (auto& c : str) {
c = std::toupper(c);
}
std::cout << str << std::endl;
return 0;
}
```
2. 转换为小写字母:
```cpp
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string str = "Hello World!";
for (auto& c : str) {
c = std::tolower(c);
}
std::cout << str << std::endl;
return 0;
}
```
这些代码中使用了`std::toupper`和`std::tolower`函数来实现大小写转换。它们可以应用于单个字符,并通过循环遍历整个字符串来实现字符串的大小写转换。
c++string大小写转换
要将 C++ 字符串的大小写转换,可以使用标准库中的函数 `std::transform` 和 `std::tolower` 或 `std::toupper`。下面是一个示例代码,展示如何将字符串转换为大写或小写:
```cpp
#include <iostream>
#include <algorithm>
#include <string>
int main() {
std::string str = "Hello World!";
// 转换为小写
std::transform(str.begin(), str.end(), str.begin(), [](unsigned char c) { return std::tolower(c); });
std::cout << str << std::endl;
// 转换为大写
std::transform(str.begin(), str.end(), str.begin(), [](unsigned char c) { return std::toupper(c); });
std::cout << str << std::endl;
return 0;
}
```
输出结果为:
```
hello world!
HELLO WORLD!
```
注意,这里使用了 `std::transform` 函数来对字符串中的每个字符进行转换。Lambda 表达式 `[](unsigned char c) { return std::tolower(c); }` 用于将字符转换为小写,而 `[](unsigned char c) { return std::toupper(c); }` 则用于将字符转换为大写。
相关推荐
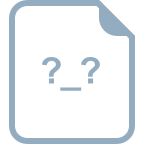
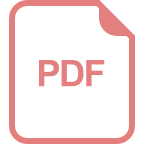












