c++for循环大小写转换
时间: 2024-03-30 17:31:55 浏览: 20
在C++中,可以使用for循环来实现大小写转换。具体的实现方式如下:
```cpp
#include <iostream>
#include <cctype>
int main() {
std::string str = "Hello World";
for (int i = 0; i < str.length(); i++) {
if (std::islower(str[i])) {
str[i] = std::toupper(str[i]);
} else if (std::isupper(str[i])) {
str[i] = std::tolower(str[i]);
}
}
std::cout << str << std::endl;
return 0;
}
```
上述代码中,我们使用了`std::islower`函数和`std::toupper`函数来将小写字母转换为大写字母,使用了`std::isupper`函数和`std::tolower`函数来将大写字母转换为小写字母。通过遍历字符串的每个字符,判断其是否为小写或大写字母,并进行相应的转换操作。
相关问题
c++ string 大小写转换
你可以使用C++的标准库中的函数来进行字符串大小写转换。下面是一些示例代码:
1. 转换为大写字母:
```cpp
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string str = "Hello World!";
for (auto& c : str) {
c = std::toupper(c);
}
std::cout << str << std::endl;
return 0;
}
```
2. 转换为小写字母:
```cpp
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string str = "Hello World!";
for (auto& c : str) {
c = std::tolower(c);
}
std::cout << str << std::endl;
return 0;
}
```
这些代码中使用了`std::toupper`和`std::tolower`函数来实现大小写转换。它们可以应用于单个字符,并通过循环遍历整个字符串来实现字符串的大小写转换。
c++中大小写字母串转换
在C++中,可以使用以下几种方法来进行大小写字母串的转换:
1. 使用标准库函数:
- `toupper(char)`:将一个小写字母转换为大写字母。
- `tolower(char)`:将一个大写字母转换为小写字母。
如果要对整个字符串进行转换,可以使用循环遍历字符串的每个字符,并对每个字符进行大小写转换。
例如:
```cpp
#include <iostream>
#include <cctype>
using namespace std;
int main() {
string str = "Hello World";
for (int i = 0; i < str.length(); i++) {
if (islower(str[i])) {
str[i] = toupper(str[i]);
} else if (isupper(str[i])) {
str[i] = tolower(str[i]);
}
}
cout << "转换后的字符串: " << str << endl;
return 0;
}
```
2. 使用位运算:
- 大写字母转换为小写字母:将字符的ASCII码值加上32。
- 小写字母转换为大写字母:将字符的ASCII码值减去32。
如果要对整个字符串进行转换,可以使用循环遍历字符串的每个字符,并对每个字符进行大小写转换。
例如:
```cpp
#include <iostream>
using namespace std;
int main() {
string str = "Hello World";
for (int i = 0; i < str.length(); i++) {
if (str[i] >= 'a' && str[i] <= 'z') {
str[i] = str[i] - 32;
} else if (str[i] >= 'A' && str[i] <= 'Z') {
str[i] = str[i] + 32;
}
}
cout << "转换后的字符串: " << str << endl;
return 0;
}
```
无论使用哪种方法,都可以实现大小写字母串的转换。具体使用哪种方法,可以根据实际需求和个人偏好进行选择。
相关推荐
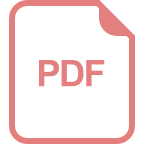
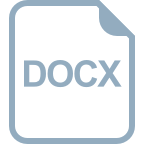












