c语言运行自动售货机查询价格
时间: 2024-09-29 15:14:40 浏览: 29
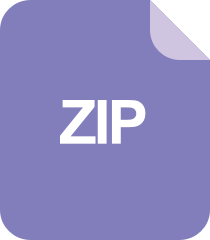
C语言模拟自动售货机系统源码.zip
在C语言中模拟一个自动售货机查询商品价格的基本程序会涉及几个关键步骤:
1. 定义产品结构体:创建一个名为`Product`的结构体,包含商品名`name`和对应的价格`price`。
```c
typedef struct {
char name[50];
float price;
} Product;
```
2. 创建产品数组:初始化一个包含若干产品的数组,每个产品都有名称和价格。
```c
Product products[] = {
{"饮料", 3.50},
{"零食", 2.00},
// 其他商品...
};
```
3. 查询功能函数:编写一个函数`getPriceByName(char *name)`,接受用户输入的商品名称作为参数,遍历产品数组查找匹配项并返回价格。
```c
float getPriceByName(char *name) {
for (int i = 0; i < sizeof(products) / sizeof(products[0]); i++) {
if (!strcmp(name, products[i].name)) {
return products[i].price;
}
}
return -1; // 商品未找到,返回错误码
}
```
4. 用户界面:创建主函数,获取用户输入的名称,然后调用`getPriceByName`函数显示价格。
```c
#include <stdio.h>
#include <string.h>
int main() {
char input[50];
printf("请输入你要查询的商品名称:");
fgets(input, sizeof(input), stdin); // 注意去掉末尾的换行符
input[strlen(input) - 1] = '\0'; // 去除fgets带来的多余字符
float price = getPriceByName(input);
if (price != -1)
printf("该商品的价格是:%f元\n", price);
else
printf("抱歉,找不到此商品。\n");
return 0;
}
```
阅读全文
相关推荐
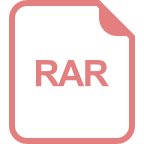
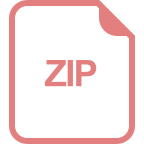
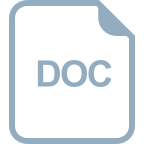
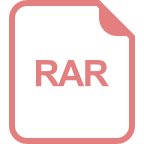
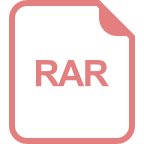
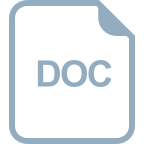
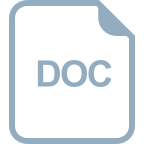

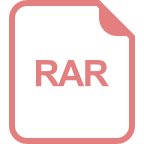
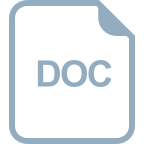
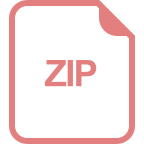
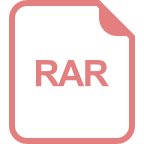
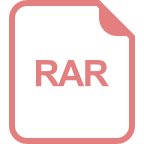
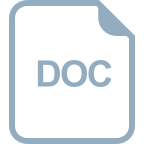
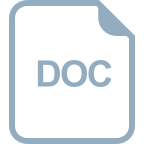

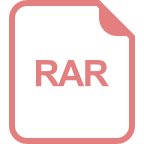