word2vec怎么预训练词向量
时间: 2023-12-14 17:32:18 浏览: 95
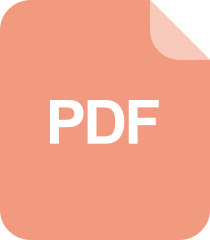
在python下实现word2vec词向量训练与加载实例

根据提供的引用内容,我们可以使用gensim中的word2vec API来预训练词向量。下面是一个简单的示例代码:
```python
from gensim.models import Word2Vec
sentences = [["cat", "say", "meow"], ["dog", "say", "woof"]]
model = Word2Vec(sentences, min_count=1)
```
在这个示例中,我们使用了两个句子来训练模型,每个句子都是一个词列表。min_count参数指定了一个词在语料库中出现的最小次数,如果一个词出现的次数小于这个值,那么它将被忽略。训练完成后,我们可以使用model.wv来访问词向量,例如:
```python
vector = model.wv['cat']
```
这将返回一个长度为100的词向量,其中100是我们在训练时指定的向量维度。我们还可以使用model.wv.most_similar()方法来查找与给定词最相似的词,例如:
```python
similar_words = model.wv.most_similar('cat')
```
这将返回一个包含最相似词及其相似度得分的元组列表。
阅读全文
相关推荐

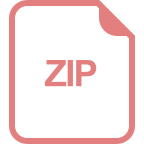
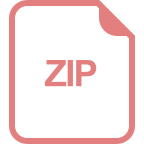

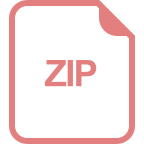
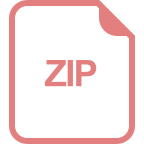
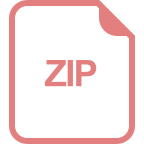






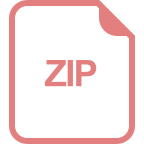
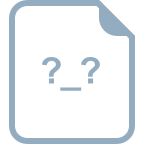
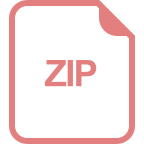