在C语言中 用两个开关控制红黄蓝绿四盏灯 两个开关同时打开灯全部亮 开关一打开开关二闭合四个灯红黄亮 ,开关二打开开关一闭合蓝绿亮。用c语言程序怎么写
时间: 2024-12-10 13:45:17 浏览: 10
在C语言中,我们可以使用布尔变量来模拟开关的状态,并利用数组来表示灯的状态。由于题目描述的情况可以用简单的逻辑条件来表示,下面是一个简单的示例程序,假设我们有两个开关`switch1`和`switch2`以及四个灯`light[]`:
```c
#include <stdio.h>
#define NUM_LIGHTS 4
typedef enum { RED = 0, GREEN, BLUE, YELLOW } Light;
int main() {
int switch1 = 0; // 0 表示关闭,1 表示开启
int switch2 = 0;
Light light[NUM_LIGHTS] = {RED, RED, RED, RED}; // 初始所有灯都是红色
// 更新灯状态
void updateLights() {
if (switch1) {
light[0] = (light[0] == RED) ? GREEN : RED; // 如果开关1打开,第一盏灯切换颜色
}
if (switch2 && !switch1) {
light[1] = (light[1] == RED) ? GREEN : RED; // 如果只有开关2打开,第二盏灯切换
}
if (!switch1 && switch2) {
light[2] = (light[2] == RED) ? BLUE : RED; // 同理,第三盏灯
}
if (switch1 && switch2) {
light[3] = RED; // 如果两开关都开,第四盏保持红色
}
}
// 打印当前灯的状态
void printLights() {
for (int i = 0; i < NUM_LIGHTS; i++) {
printf("Light %d: ", i);
switch (light[i]) {
case RED: printf("Red\n"); break;
case GREEN: printf("Green\n"); break;
case BLUE: printf("Blue\n"); break;
case YELLOW: printf("Yellow\n"); break;
}
}
}
updateLights(); // 初次更新灯状态
printLights();
// 模拟操作开关
switch1 = 1; // 操作开关1
updateLights();
printLights();
switch2 = 1; // 操作开关2
updateLights();
printLights();
return 0;
}
```
在这个程序里,我们首先初始化了所有的灯为红色,然后定义了一个`updateLights`函数来根据开关的状态更新灯的颜色。在主循环中,你可以模拟开关的操作并打印灯的状态。
阅读全文
相关推荐
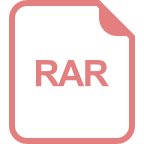
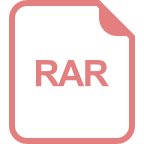
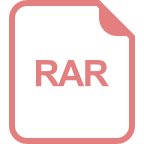















