c# 写一段程序 向 http://121.36.111.13:8021/otherRoute/getWorkerList 发送json
时间: 2024-10-08 16:01:08 浏览: 26
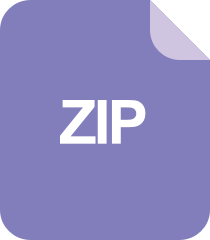
C# Http(Get/Post)以及Json解析源码
在 C# 中,你可以使用 HttpClient 类来自定义发送 HTTP 请求。这里是一个简单的示例,展示如何向给定地址发送一个 JSON 数据的 GET 请求:
```csharp
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
public class Program
{
private const string Url = "http://121.36.111.13:8021/otherRoute/getWorkerList";
private static readonly HttpClient client = new HttpClient();
public static async Task Main()
{
// 创建一个 JSON 对象或数据字典
var jsonContent = new { Name = "John Doe", ID = 12345 };
// 将 JSON 转换为字符串
var jsonString = Newtonsoft.Json.SerializeObject(jsonContent);
try
{
// 设置请求头为 JSON 格式
var requestJson = new StringContent(jsonString, Encoding.UTF8, "application/json");
HttpResponseMessage response = await client.GetAsync(Url, HttpCompletionOption.ResponseHeadersRead, requestJson);
if (response.IsSuccessStatusCode)
{
Console.WriteLine($"HTTP Status Code: {response.StatusCode}");
string responseBody = await response.Content.ReadAsStringAsync();
Console.WriteLine("Response Body: " + responseBody);
}
else
{
Console.WriteLine($"Request failed with status code: {response.StatusCode}");
}
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred: {ex.Message}");
}
}
}
```
在这个例子中,我们首先创建了一个 JSON 字符串,然后将其作为 `HttpClient` 的内容发送。注意,你需要安装 `Newtonsoft.Json` 库来序列化 JSON,可以使用 NuGet 包管理器添加。
阅读全文
相关推荐
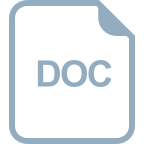
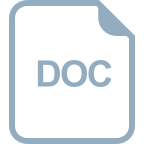

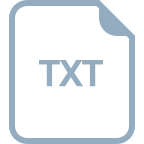
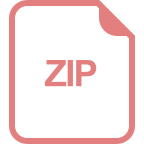
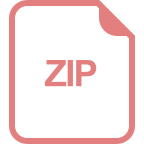
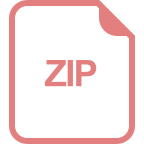
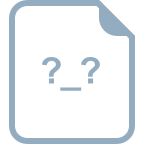
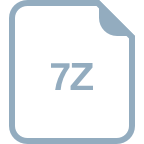
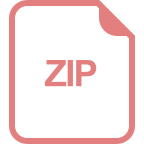
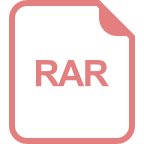
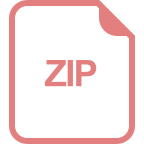
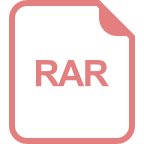
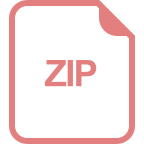
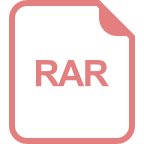
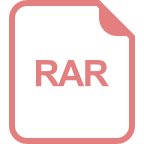
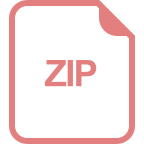
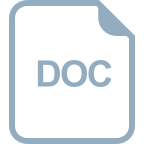