写出一个与矩阵链最优的计算顺序有关的Java代码
时间: 2024-10-11 20:13:06 浏览: 21
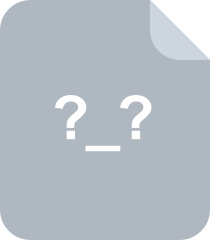
算法导论15.2-1矩阵链最优括号化方案

矩阵链乘法(Matrix Chain Multiplication, MCM)是一个经典的问题,它涉及到计算一系列矩阵相乘的最有效顺序,以最小化所需的运算次数。这里给出一个简单的递归算法的Java实现:
```java
public class MatrixChainOrder {
// 定义一个常量数组用于存储中间结果
private static int[] dp;
public static int matrixChainOrder(int[][] mat, int n) {
if (n == 1) return 0; // 如果只有一个矩阵,无需乘法
dp = new int[n]; // 初始化动态规划数组
// 从规模2到n遍历,尝试所有可能的分割
for (int size = 2; size < n; size++) {
for (int l = 1; l < n - size + 1; l++) {
int r = l + size - 1;
dp[l] = Math.min(dp[l], dp[l + 1] + dp[r] + cost(mat, l, r, size));
}
}
return dp[1]; // 返回最小成本,即最终结果
}
private static int cost(int[][] mat, int i, int j, int k) {
// 计算两个子矩阵相乘所需的成本(即额外的乘法次数)
return mat[i - 1][k - 1].length * mat[k][j].length;
}
public static void main(String[] args) {
int[][] mat = { {1, 2}, {3, 4}, {5, 6}, {7, 8} }; // 例子中的矩阵
int n = mat.length;
System.out.println("Minimum number of scalar multiplications required is: " + matrixChainOrder(mat, n));
}
}
```
这个代码首先定义了一个动态规划函数`matrixChainOrder`,其中`dp`数组记录了以每个子矩阵开始和结束的最优乘法序列的最小成本。然后通过两个嵌套循环遍历所有可能的分割,并找到最小成本。最后,在`main`函数中,我们提供一个矩阵实例并计算其最优乘法顺序。
阅读全文
相关推荐
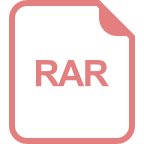
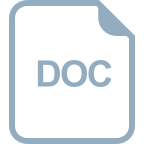
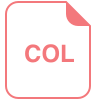
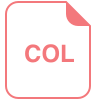
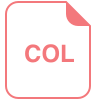
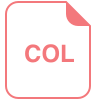
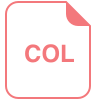
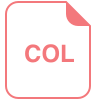
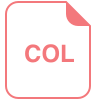
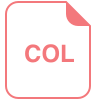
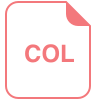
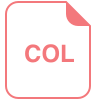
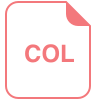
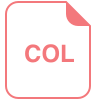
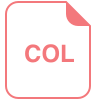
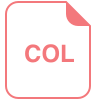