winform使用SharpGL实现三维坐标系
时间: 2024-09-23 16:05:25 浏览: 116
WinForm是Windows应用程序框架的一部分,主要用于创建图形用户界面。 SharpGL是一个用于.NET平台的OpenGL库,它提供了一个简单的方式来利用OpenGL在Windows Forms应用中实现3D渲染,包括三维坐标系。
在WinForms中使用SharpGL实现三维坐标系,你可以按照以下步骤操作:
1. 引入依赖:首先需要在项目中添加SharpGL的NuGet包,以便使用其API。
```csharp
using System.Windows.Forms;
using OpenGL;
```
2. 设置OpenGL上下文:在Form的Load事件中,初始化OpenGL窗口并设置渲染模式。
```csharp
protected override void OnCreateControl()
{
base.OnCreateControl();
GLControl glControl = new GLControl();
glControl.Dock = DockStyle.Fill;
// 初始化OpenGL context
glControl.MakeCurrent();
GL.glClearColor(0f, 0f, 0f, 1f); // 设置背景颜色
glControl.GLContext.ClearCapabilities = ClearBufferMask.ColorBufferBit;
}
// 等待窗口关闭
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
GLControl glControl = (GLControl)sender;
glControl.Dispose(); // 关闭OpenGL上下文
}
```
3. 绘制坐标轴:通过GL.Draw Axis()函数或自定义顶点数组来绘制X、Y和Z轴。
```csharp
void DrawAxis()
{
float[] vertices = {
-1, 0, 0,
1, 0, 0,
0, 1, 0,
0, -1, 0,
0, 0, 1,
0, 0, -1
};
int[] indices = {
0, 1, 2, // X轴
2, 3, 0, // Y轴
4, 5, 6, // Z轴
};
// 使用顶点缓冲区对象(Vertex Buffer Object,VBO)加速渲染
//...
GL.DrawArrays(PrimitiveType.Lines, 0, indices.Length);
}
```
4. 在Form的Paint事件中调用DrawAxis()来显示坐标系,并定期更新视图。
```csharp
private void Form1_Paint(object sender, PaintEventArgs e)
{
glControl.SwapBuffers();
DrawAxis();
}
```
阅读全文
相关推荐








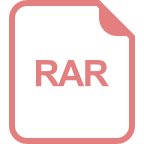









