Python timer
时间: 2023-11-05 08:17:40 浏览: 40
Python中的threading模块提供了一个Timer类,用于在指定时间后执行一个函数。Timer类继承自Thread类,因此可以在后台运行。Timer对象的构造函数需要两个参数:第一个参数是等待的时间(以秒为单位),第二个参数是要执行的函数。例如,以下代码将在5秒后打印一条消息:
import threading
def print_message():
print("Hello, world!")
timer = threading.Timer(5, print_message)
timer.start()
在上面的代码中,我们创建了一个名为timer的Timer对象,它将在5秒后调用print_message函数。调用timer.start()方法启动计时器。当计时器到期时,它将调用print_message函数并打印一条消息。
相关问题
Python timer
Python中的timer是一种用于定时执行特定任务的机制。通过使用线程来实现定时器,可以在指定的时间间隔后触发相应的事件。在实际应用中,定时器经常被用于执行周期性的任务或触发特定的事件。
例如,使用Python中的threading模块,我们可以创建一个定时器并指定一个函数作为定时器到期时要执行的任务。在定时器的构造函数中,我们可以指定时间间隔(以秒为单位)和要执行的函数。当定时器启动后,它将在指定的时间间隔后触发函数执行。
然而需要注意的是,timer只会触发一次任务执行,并且程序会在任务执行完毕后结束。如果我们希望定时器周期性地执行任务,我们需要在任务执行完毕后重新启动定时器。如果我们想要停止定时器的运行,可以使用stop()方法来停止定时器的执行。如果我们尝试启动一个已经在运行的定时器,将会引发TimerError异常。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [python timer使用-Python timer定时器两种常用方法解析](https://blog.csdn.net/weixin_37988176/article/details/109414576)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [python timer怎么用_python定时器(Timer)用法简单实例](https://blog.csdn.net/weixin_39608748/article/details/110403551)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
python timer
Here's an example of a timer in Python:
```python
import time
def timer(seconds):
start_time = time.time()
while time.time() - start_time < seconds:
pass
print("Time's up!")
# Example usage: timer for 5 seconds
timer(5)
```
This code uses the `time` module to measure the time passed and waits until the specified number of seconds has elapsed before printing "Time's up!". This can be used for various purposes, such as creating a game timer or measuring the execution time of a function.
相关推荐
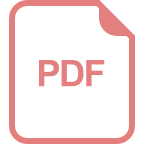













