游程编码用c语言实现
时间: 2024-10-25 18:05:14 浏览: 18
游程编码是一种数据压缩算法,用于表示图像、图形等二进制数据,通过替换连续的像素值序列为一个单独的数值来节省存储空间。在C语言中实现游程编码,可以分为以下几个步骤:
1. **读取数据**:首先需要读取图像数据,将其转换成一维数组,每个元素代表一个像素。
2. **分块处理**:将像素值分割成若干个连续的块,因为游程编码是对连续像素值的编码。
3. **计数构建码字**:对于每个像素块,统计其出现的次数(也就是该颜色的像素数目),然后创建一个码字,通常是一个整数,它等于块的开始位置加上块内的像素数目。
4. **编码存储**:使用位操作或自定义的数据结构(如链表或数组)来存储这些码字,较短的码字可以用更少的位来表示,提高了整体的压缩效率。
5. **解码还原**:读取存储的码字,根据码字长度和起始位置,恢复原始的像素值序列。
下面是一个简单的游程编码示例(仅做演示,实际应用需要考虑性能优化):
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int start;
int count;
} RunLength;
void encode(int* pixels, int width, int height, RunLength* encoded) {
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
// 检查是否与前一个像素相同
if (x > 0 && pixels[x] == pixels[x - 1]) {
encoded->count++;
} else {
encoded->start = pixels[x];
encoded->count = 1;
}
}
// Move to next row
pixels += width;
}
}
void decode(RunLength* encoded, int* output, int width, int height) {
int current_pos = 0;
for (int y = 0; y < height; y++) {
for (int i = 0; i < encoded->count; i++) {
output[current_pos++] = encoded->start;
}
encoded++; // Move to next run
}
}
int main() {
int width = 10;
int height = 10;
int* pixels = malloc(width * height);
// 填充像素...
RunLength encoded[width * height];
encode(pixels, width, height, encoded);
// 写入或解码到文件...
free(pixels);
return 0;
}
```
阅读全文
相关推荐
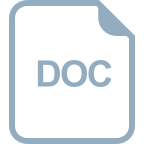
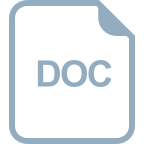
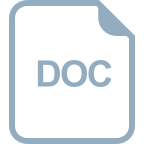
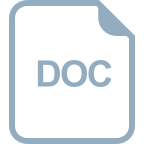
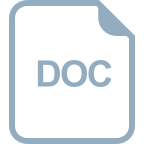
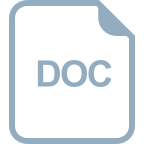


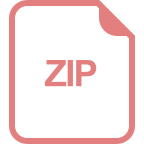



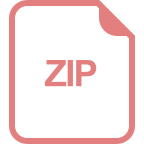
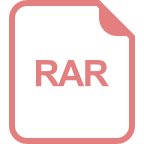
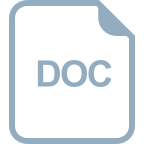
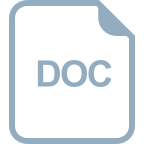


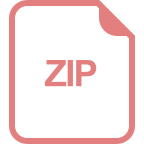