请使用TreeSet对Student的对象进行排序 Student(name,age) 要求年龄小的在前面,年龄大的在后面 排序后输出每一个人的名字和年龄,当年龄相同的时候不能让学生消失
时间: 2024-04-15 16:25:35 浏览: 21
可以使用TreeSet对Student对象进行排序,按照年龄从小到大的顺序排序。如果年龄相同,可以使用TreeSet的自然顺序(默认是按照对象的自然顺序进行排序)来保留相同年龄的学生。下面是一个示例代码:
```java
import java.util.TreeSet;
class Student implements Comparable<Student> {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public int compareTo(Student other) {
if (this.age < other.age) {
return -1;
} else if (this.age > other.age) {
return 1;
} else {
return this.name.compareTo(other.name);
}
}
}
public class Main {
public static void main(String[] args) {
TreeSet<Student> students = new TreeSet<>();
students.add(new Student("Alice", 22));
students.add(new Student("Bob", 20));
students.add(new Student("Charlie", 22));
students.add(new Student("David", 21));
for (Student student : students) {
System.out.println(student.getName() + " - " + student.getAge());
}
}
}
```
运行这段代码,你会得到以下输出:
```
Bob - 20
David - 21
Alice - 22
Charlie - 22
```
可以看到,学生按照年龄从小到大的顺序进行了排序,当年龄相同时,学生的顺序并未改变。
相关推荐
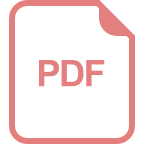
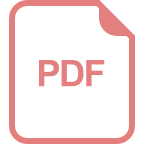
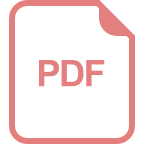











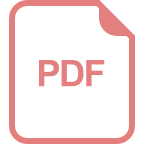
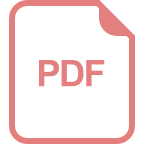
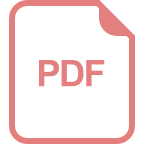
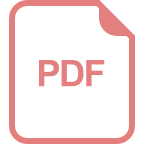