java使用http请求如何form表单形式
时间: 2024-10-18 14:03:36 浏览: 27
在Java中,通常使用HttpURLConnection、HttpClient或者其他第三方库如Apache HttpClient或者OkHttp来进行HTTP请求。如果你需要发送包含表单数据的POST请求,可以按照以下步骤操作:
1. 导入所需的库(例如使用HttpURLConnection):
```java
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
```
2. 创建URL实例并连接到服务器:
```java
URL url = new URL("http://example.com/api/endpoint");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST"); // 设置请求方法为POST
```
3. 将表单数据转化为字节数组(假设有一个Map<String, String> formData):
```java
String formEncodedData = "";
for (Map.Entry<String, String> entry : formData.entrySet()) {
formEncodedData += entry.getKey() + "=" + entry.getValue() + "&";
}
formEncodedData = formEncodedData.substring(0, formEncodedData.length() - 1); // 去掉最后一个 &
```
4. 设置请求头,指定Content-Type为"application/x-www-form-urlencoded":
```java
connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
```
5. 写入表单数据:
```java
OutputStream os = connection.getOutputStream();
os.write(formEncodedData.getBytes()); // 注意编码可能需要设置为UTF-8
os.flush();
os.close();
```
6. 接收响应并处理结果:
```java
int responseCode = connection.getResponseCode();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println(response.toString());
} else {
// 处理错误响应
}
```
阅读全文
相关推荐
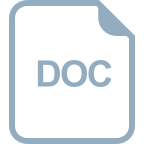
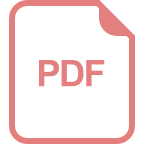
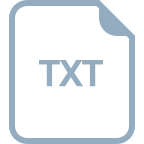
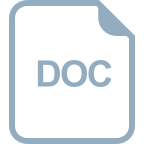
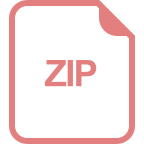
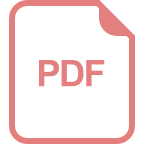
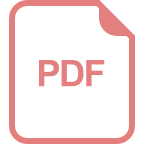
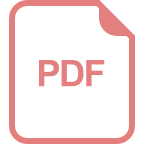
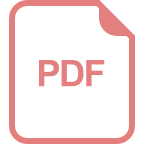
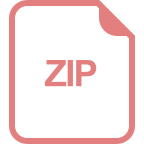
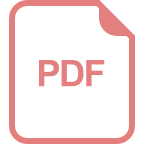
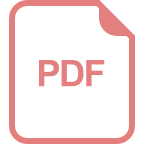
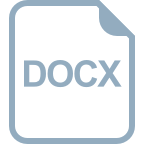
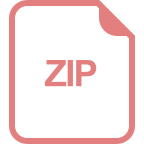
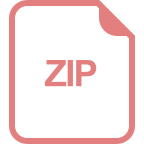
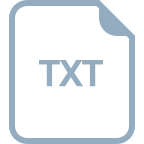
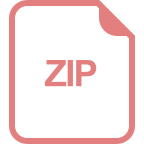