俄罗斯方块图形库代码
时间: 2023-10-26 19:08:33 浏览: 130
这里是一个使用 Python 和 Pygame 实现的俄罗斯方块图形库的代码示例:
```python
import pygame
import random
# 定义方块的形状
shapes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6],
[6, 6]],
[[7, 7, 7, 7]]
]
# 定义方块的颜色
colors = [
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(128, 128,128)
]
class Tetris:
def __init__(self):
pygame.init()
pygame.display.set_caption("Tetris")
self.width = 320
self.height = 480
self.screen = pygame.display.set_mode((self.width, self.height))
self.clock = pygame.time.Clock()
self.block_size = min(self.width // 10, self.height // 20)
self.font = pygame.font.SysFont("Arial", self.block_size * 2 // 3)
self.score = 0
self.board = [[0] * 10 for _ in range(20)]
self.current_shape = None
self.current_x = 0
self.current_y = 0
self.next_shape = None
self.game_over = False
def new_shape(self):
self.current_shape = self.next_shape or random.choice(shapes)
self.next_shape = random.choice(shapes)
self.current_x = 5 - len(self.current_shape[0]) // 2
self.current_y = 0
if self.check_collision(self.current_shape, self.current_x, self.current_y):
self.game_over = True
def check_collision(self, shape, x, y):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] and (y + i >= len(self.board) or x + j < 0 or x + j >= len(self.board[i]) or self.board[y + i][x + j]):
return True
return False
def merge_board(self):
for i in range(len(self.current_shape)):
for j in range(len(self.current_shape[i])):
if self.current_shape[i][j]:
self.board[self.current_y + i][self.current_x + j] = self.current_shape[i][j]
def remove_lines(self):
lines_removed = 0
new_board = [[0] * 10 for _ in range(20)]
i = len(self.board) - 1
for row in reversed(self.board):
if 0 not in row:
lines_removed += 1
else:
new_board[i] = row
i -= 1
self.board = new_board
self.score += lines_removed ** 2
def draw_block(self, x, y, color):
pygame.draw.rect(self.screen, color, (x * self.block_size, y * self.block_size, self.block_size, self.block_size))
def draw_board(self):
for i in range(len(self.board)):
for j in range(len(self.board[i])):
if self.board[i][j]:
self.draw_block(j, i, colors[self.board[i][j] - 1])
def draw_shape(self, shape, x, y):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j]:
self.draw_block(x + j, y + i, colors[shape[i][j] - 1])
def draw_text(self, text, x, y):
surface = self.font.render(text, True, (255, 255, 255))
rect = surface.get_rect()
rect.center = (x, y)
self.screen.blit(surface, rect)
def run(self):
while not self.game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if not self.check_collision(self.current_shape, self.current_x - 1, self.current_y):
self.current_x -= 1
elif event.key == pygame.K_RIGHT:
if not self.check_collision(self.current_shape, self.current_x + 1, self.current_y):
self.current_x += 1
elif event.key == pygame.K_DOWN:
if not self.check_collision(self.current_shape, self.current_x, self.current_y + 1):
self.current_y += 1
elif event.key == pygame.K_UP:
rotated_shape = [[shape[len(shape) - j - 1][i] for j in range(len(shape))] for i in range(len(shape[0]))]
if not self.check_collision(rotated_shape, self.current_x, self.current_y):
self.current_shape = rotated_shape
elif event.key == pygame.K_SPACE:
while not self.check_collision(self.current_shape, self.current_x, self.current_y + 1):
self.current_y += 1
self.screen.fill((0, 0, 0))
self.draw_board()
self.draw_shape(self.current_shape, self.current_x, self.current_y)
self.draw_text("Score: {}".format(self.score), self.width // 2, self.block_size // 2)
pygame.display.flip()
if self.check_collision(self.current_shape, self.current_x, self.current_y + 1):
self.merge_board()
self.remove_lines()
self.new_shape()
self.clock.tick(10)
pygame.quit()
if __name__ == "__main__":
game = Tetris()
game.run()
```
阅读全文
相关推荐
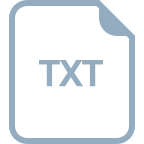
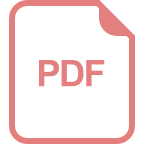
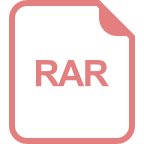
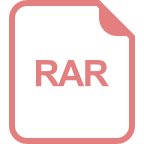
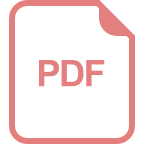
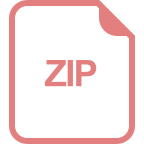
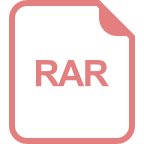
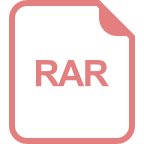
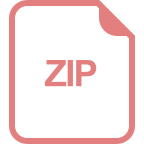
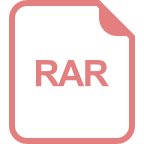
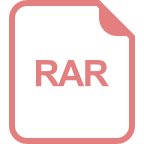
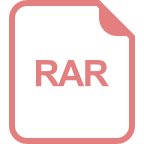
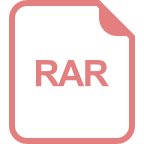
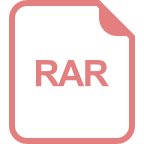
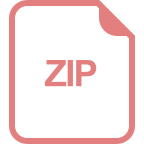
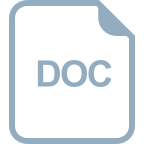