出一个python通过面部识别年龄的代码
时间: 2024-11-15 14:32:19 浏览: 3
在Python中,要实现人脸识别并估计年龄,通常我们会使用OpenCV库结合深度学习模型。一个常见的方法是利用预训练的人脸检测模型(如Haar级联分类器或Dlib库的`face_recognition_hog_face_detector`)定位人脸,然后使用预训练的面部识别模型(比如dlib或Face++ API),再结合一个预训练的年龄性别识别模型(例如VGGFace2或者MTCNN)。以下是一个简单的示例,使用dlib库进行人脸检测和age.pynt模型进行年龄估计:
```python
import cv2
from dlib import get_frontal_face_detector, facerec, load_rgb_image
from imutils import face_utils
import numpy as np
# 加载预训练的面部识别模型
detector = get_frontal_face_detector()
predictor = face_utils.shape_predictor("shape_predictor_68_face_landmarks.dat")
facerec_model = "model/agedb_30.norm.pkl"
# 预加载年龄预测模型
age_predictor = facerec(open(facerec_model, 'rb'))
def detect_and_estimate_age(image_path):
# 读取图像
img = cv2.imread(image_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = detector(gray)
ages = []
for face in faces:
# 获取脸部关键点
shape = predictor(gray, face)
shape = face_utils.shape_to_np(shape)
# 提取特征并计算年龄
face_descriptor = facerec_model.compute descriptors(face)
age, gender, prob = age_predictor.predict(face_descriptor)
# 返回年龄估计
ages.append(age)
# 可能还需要根据实际需求处理其他信息,比如打印或返回性别概率
return ages
# 使用方法
image_path = "path/to/your/image.jpg"
ages = detect_and_estimate_age(image_path)
print(f"Detected ages: {ages}")
```
请注意,这个例子假设你已经下载了所有必要的预训练模型文件(包括形状预测器、面部识别模型以及年龄预测模型)。此外,这个代码可能需要安装`imutils`, `numpy`和`dlib`库。
阅读全文
相关推荐
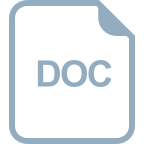
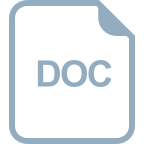
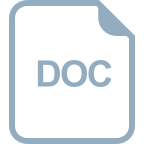

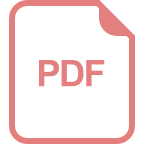
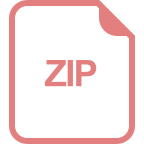
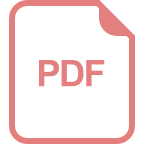
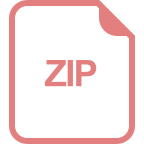
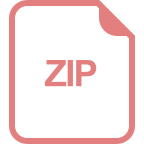
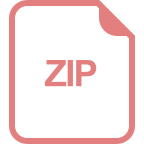
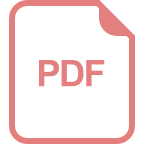
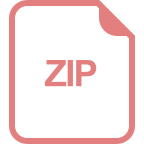
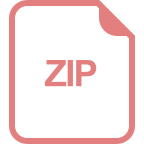
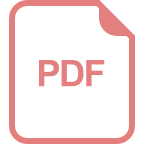
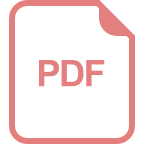
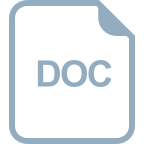
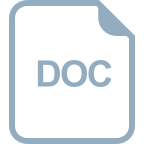
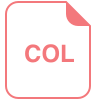