python 预测天气预报
时间: 2024-12-28 22:14:31 浏览: 3
### 使用Python实现天气预报预测
为了通过Python实现天气预报预测功能,通常会采用调用第三方气象服务API的方式获取最新的天气数据并解析展示。这不仅限于简单地抓取网页内容,而是利用结构化的API接口来获得更精准的数据。
#### 获取API密钥
大多数在线天气服务平台提供免费版和付费版本的服务,在注册账号之后能够得到一个唯一的API Key用于身份验证。此Key将在请求URL中作为参数传递给服务器端以完成授权访问[^1]。
#### 安装必要的库
对于发送HTTP请求以及处理JSON响应来说,`requests`是一个非常好用的第三方模块;而要解析返回的时间戳等信息,则可能需要用到`datetime`标准库中的函数:
```bash
pip install requests
```
#### 编写代码逻辑
下面是一份基础示例代码片段展示了如何构建这样的应用程序框架:
```python
import requests
from datetime import datetime
def get_weather(city_name, api_key):
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = f"{base_url}appid={api_key}&q={city_name}"
response = requests.get(complete_url)
data = response.json()
if data["cod"] != "404":
main_data = data['main']
weather_desc = data['weather'][0]['description']
temp_kelvin = main_data['temp'] # 温度单位为开尔文(Kelvin)
pressure = main_data['pressure']
humidity = main_data['humidity']
wind_speed = data['wind']['speed']
sunrise_time = datetime.utcfromtimestamp(data['sys']['sunrise']).strftime('%Y-%m-%d %H:%M:%S')
sunset_time = datetime.utcfromtimestamp(data['sys']['sunset']).strftime('%Y-%m-%d %H:%M:%S')
result_str = (f"City: {city_name}\n"
f"Weather Description: {weather_desc}\nTemperature (in kelvin unit): {str(temp_kelvin)}\n"
f"Atmospheric Pressure(in hPa unit): {str(pressure)}\nHumidity(%): {str(humidity)}\n"
f"Wind Speed(m/s): {str(wind_speed)}\nSunrise Time(UTC): {sunrise_time}\n"
f"Sunset Time(UTC): {sunset_time}")
return result_str
else:
return 'City Not Found'
if __name__ == "__main__":
city = input("Enter City Name : ")
key = "<Your_API_Key_Here>" # 替换成自己的OpenWeatherMap API Key
print(get_weather(city, key))
```
这段脚本实现了向用户提供输入城市名称后查询该地区当前天气状况的功能,并打印出包括温度、气压在内的多项指标值。
阅读全文
相关推荐
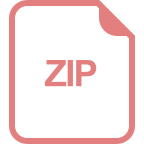
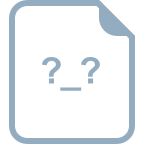
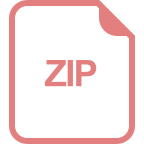
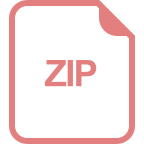
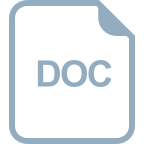
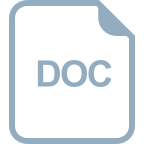
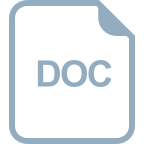
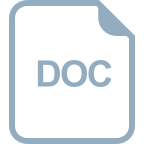









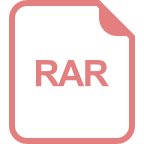