pyside6 定义一个QGraphicsView控件,显示图片,选择第一个点(加粗 颜色红色),选择下一个点的时候有一个临时线,选择多个点之后双击围成一个多边形。在开始画下一个
时间: 2024-12-11 10:32:19 浏览: 10
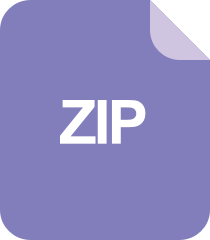
YOLOv9 基于 Pyside6 的图形化界面
在PySide6中,要创建一个`QGraphicsView`控件显示图片,并允许用户绘制并选择多个点形成多边形,你需要遵循以下步骤:
1. **设置环境**:
确保已经安装了PySide6库以及相关的图形处理库(如Qt Gui模块)。你可以通过pip安装:
```bash
pip install pyside6 PySide6-tools
```
2. **初始化QGraphicsView**:
创建一个新的`QGraphicsView`实例,绑定到一个`QGraphicsScene`,用于放置图形元素:
```python
from PySide6.QtCore import QRectF, Qt
from PySide6.QtGui import QPixmap, QPainterPath, QColor, QPen, QPainter, QGraphicsPolygonItem
from PySide6.QtWidgets import QApplication, QWidget
class MyWindow(QWidget):
def __init__(self):
super().__init__()
self.view = QGraphicsView(self)
self.scene = QGraphicsScene(self.view)
# 设置视口
self.view.setScene(self.scene)
self.view.setViewportUpdateMode(QGraphicsView.BoundingRectViewportUpdate)
```
3. **加载图片并显示**:
加载一张图片作为背景,并将其转换为`QPixmap`:
```python
pixmap = QPixmap('path_to_your_image.png')
image_item = QGraphicsPixmapItem(pixmap)
self.scene.addItem(image_item)
```
4. **处理鼠标事件**:
- 记录鼠标点击位置
- 创建`QPainterPath`实例,并添加新的点
- 更新场景中的临时线(可以用虚线表示)
5. **选择点并显示临时线**:
- `mouseMoveEvent`处理移动过程,更新临时线的位置
- 当释放鼠标(`mouseReleaseEvent`)时:
- 检查是否选择了两个以上的点
- 如果是,将所有点连接起来形成`QPainterPath`
- 创建`QGraphicsPolygonItem`并设置颜色和粗细
- 添加到场景中
- 清除临时线
示例代码片段:
```python
class PointSelection(QGraphicsPolygonItem):
def __init__(self, points, color=QColor("red"), width=2):
super().__init__()
self.path = QPainterPath()
for point in points:
self.path.lineTo(point)
self.setPen(QPen(color, width))
def paint(self, painter, option, widget=None):
painter.drawPath(self.path)
# ... (继续处理鼠标事件部分)
def mouseMoveEvent(event):
if not self.last_point:
return
temp_line = QGraphicsLineItem(self.last_point, event.pos())
temp_line.setPen(QPen(QColor(0, 0, 0, 100), 1)) # 虚线临时线
self.scene.addItem(temp_line)
def mouseReleaseEvent(event):
global last_points
if not last_points or len(last_points) < 2:
return
polygon = PointSelection(last_points)
self.scene.addItem(polygon)
# 清理临时线
for item in self.scene.items():
if isinstance(item, QGraphicsLineItem) and item.pen().color().lightness() > 100:
self.scene.removeItem(item)
last_point = None
last_points = []
app = QApplication([])
window = MyWindow()
window.show()
app.exec_()
```
阅读全文
相关推荐
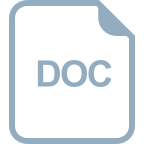
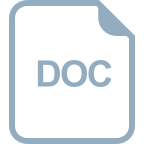
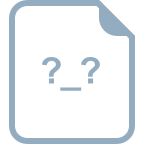














