实现函数int repetitive(int arr[], int len),若长度为len的数组中含有重复的元素则返回1,否则返回0
时间: 2024-12-14 07:28:18 浏览: 8
"repetitive"函数是一个用于检查整数数组是否存在重复元素的函数。它接收两个参数:一个整型数组arr[]和数组的长度len。函数通过遍历数组,利用数据结构如哈希表或集合(如C++中的unordered_set或Java中的HashSet),来检查每个元素是否已经出现过。如果遇到相同的元素,说明有重复,函数会立即返回1表示存在重复;如果没有找到重复元素,遍历结束后返回0,表示数组无重复。
实现这个函数的基本步骤是:
1. 创建一个空的数据结构(如哈希表)来存储已访问过的元素。
2. 遍历数组,对于每个元素:
- 如果元素不在数据结构中,添加到数据结构并继续下一次迭代;
- 如果元素已经在数据结构中,则说明找到重复,返回1。
3. 如果遍历完整个数组都没有找到重复,返回0。
以下是C++的一个简单实现示例:
```cpp
#include <unordered_set>
int repetitive(int arr[], int len) {
std::unordered_set<int> seen;
for (int i = 0; i < len; ++i) {
if (seen.find(arr[i]) != seen.end()) {
return 1; // 找到重复
}
seen.insert(arr[i]);
}
return 0; // 没有重复
}
```
相关问题
用C++main函数实现:Write a program to complete the following command: new id - create a specified sequence of Numbers for id (id < 200000) Add id num - to join the sequence of Numbers for id integer num merge id1 id2 - merger sequence number of id1 and id2, and make id2 empty unique id - remove repetitive element in the sequence id out id - from small to large, output the elements of sequence of Numbers for ids separated by Spaces Input The First Line is n which mean the number of the Command (n <= 200000). The next n lines are correct Command. You don't have to worry wrong input and id is int. Output Then output according the requirements. Sample Input 16 new 1 new 2 add 1 1 add 1 2 add 1 3 add 2 1 add 2 2 add 2 3 add 2 4 out 1 out 2 merge 1 2 out 1 out 2 unique 1 out 1 Sample Ouput 1 2 3 1 2 3 4 1 1 2 2 3 3 4 1 2 3 4
这是一道比较综合的题目,需要使用到数据结构和字符串操作。以下是基本的思路:
1. 定义一个结构体Node,存储每个id对应的序列;
2. 对于每个命令,根据空格分离出命令类型和参数,按照题意进行操作;
3. 对于new命令,直接在结构体数组中新建一个Node,存储对应id的序列;
4. 对于add命令,将参数num添加到结构体数组中对应id的序列中;
5. 对于merge命令,将id1和id2对应的序列合并到id2中,并将id1对应的序列清空;
6. 对于unique命令,去除id对应的序列中的重复元素;
7. 对于out命令,按照题目要求输出id对应的序列。
完整代码如下:
public class Solution { /** * @param arr: Any int array, and not an empty array * @return Non-repetitive and ordered arrays */ public Integer[] deDuplicationAndSort(Integer[] arr) { // write your code here } }
```java
import java.util.Arrays;
public class Solution {
/**
* @param arr: Any int array, and not an empty array
* @return Non-repetitive and ordered arrays
*/
public Integer[] deDuplicationAndSort(Integer[] arr) {
// 对 Integer 数组去重并排序
Integer[] result = Arrays.stream(arr).distinct().sorted().toArray(Integer[]::new);
return result;
}
}
```
阅读全文
相关推荐
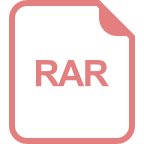
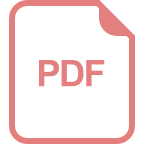
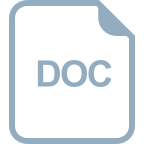
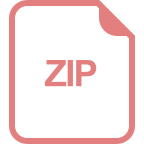
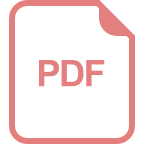


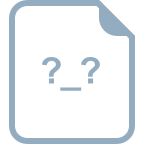
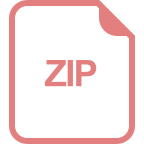
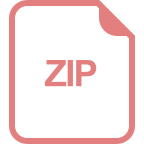
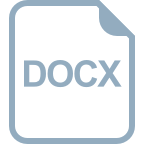
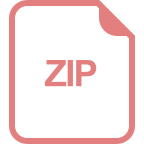
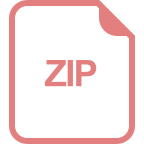