Write a program to complete the following command: new id - create a specified sequence of Numbers for id (id < 200000) Add id num - to join the sequence of Numbers for id integer num merge id1 id2 - merger sequence number of id1 and id2, and make id2 empty unique id - remove repetitive element in the sequence id out id - from small to large, output the elements of sequence of Numbers for ids separated by Spaces Input The First Line is n which mean the number of the Command (n <= 200000). The next n lines are correct Command. You don't have to worry wrong input and id is int. Output Then output according the requirements.
时间: 2024-02-14 11:15:56 浏览: 21
Sure, I can help you write a program to complete the given commands. Here's the Python code:
```
n = int(input())
sequences = {}
def new_sequence(id):
if id < 200000:
sequences[id] = []
def add_number(id, num):
if id in sequences:
sequences[id].append(num)
def merge_sequences(id1, id2):
if id1 in sequences and id2 in sequences:
sequences[id2] = sorted(sequences[id1] + sequences[id2])
sequences[id1] = []
def unique_elements(id):
if id in sequences:
sequences[id] = list(set(sequences[id]))
def output_elements(id):
if id in sequences:
print(' '.join(map(str, sorted(sequences[id]))))
for i in range(n):
command = input().split()
if command[0] == 'new':
new_sequence(int(command[1]))
elif command[0] == 'add':
add_number(int(command[1]), int(command[2]))
elif command[0] == 'merge':
merge_sequences(int(command[1]), int(command[2]))
elif command[0] == 'unique':
unique_elements(int(command[1]))
elif command[0] == 'out':
output_elements(int(command[1]))
```
The program first reads in the number of commands, then initializes an empty dictionary called `sequences` to store the sequences of numbers for each ID.
The program then defines five functions to handle each of the commands.
- `new_sequence` creates a new empty sequence for the specified ID.
- `add_number` adds a number to the sequence for the specified ID.
- `merge_sequences` merges the sequences for two specified IDs by concatenating them and sorting the result into the sequence for the second ID.
- `unique_elements` removes duplicate elements from the sequence for the specified ID.
- `output_elements` sorts the elements in the sequence for the specified ID and outputs them separated by spaces.
Finally, the program reads in each command, parses it into its components, and calls the appropriate function based on the command keyword.
I hope this helps! Let me know if you have any questions.
相关推荐
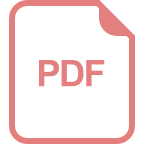
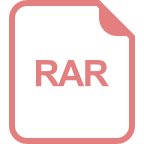
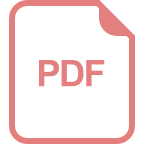




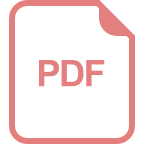
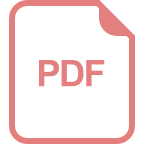
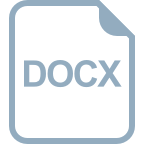
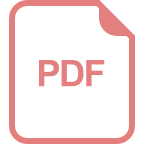
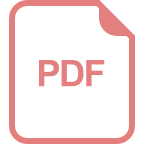
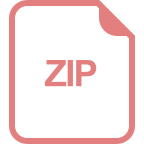
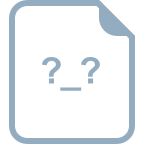
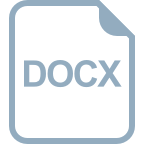
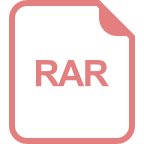
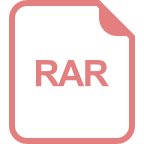
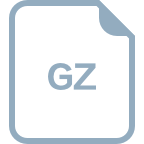