java excel去除空列
时间: 2025-01-07 09:11:36 浏览: 2
### 使用Apache POI移除Excel文件中的空列
当处理Excel文件并希望移除非空数据列时,可以利用Apache POI库来实现这一目标。下面是一个具体的解决方案:
为了检测和删除工作表内的空白列,程序会遍历每一行的数据单元格,并统计每列中非空单元的数量。如果某列内所有单元均为空,则该列被标记为可删除。
```java
import org.apache.poi.ss.usermodel.*;
import java.io.FileInputStream;
import java.util.ArrayList;
import java.util.List;
public class ExcelColumnRemover {
public static void main(String[] args) throws Exception {
String filePath = "example.xlsx";
try (FileInputStream fis = new FileInputStream(filePath);
Workbook workbook = WorkbookFactory.create(fis)) {
Sheet sheet = workbook.getSheetAt(0); // 获取第一个sheet
List<Integer> columnsToRemove = findEmptyColumns(sheet);
removeColumns(sheet, columnsToRemove);
// Save the changes to a new file.
FileOutputStream out = new FileOutputStream("output.xlsx");
workbook.write(out);
out.close();
}
}
private static List<Integer> findEmptyColumns(Sheet sheet) {
int lastRowNum = sheet.getLastRowNum();
boolean[] isEmptyCol = new boolean[sheet.getRow(0).getLastCellNum()];
for (int colIndex = 0; colIndex < isEmptyCol.length; ++colIndex) {
boolean isCurrentColEmpty = true;
for (int rowIndex = 0; rowIndex <= lastRowNum && isCurrentColEmpty; ++rowIndex) {
Row row = sheet.getRow(rowIndex);
if (row != null) {
Cell cell = row.getCell(colIndex);
if (cell != null && !isEmpty(cell)) {
isCurrentColEmpty = false;
}
}
}
isEmptyCol[colIndex] = isCurrentColEmpty;
}
List<Integer> result = new ArrayList<>();
for (int i = 0; i < isEmptyCol.length; ++i) {
if (isEmptyCol[i]) {
result.add(i);
}
}
return result;
}
private static boolean isEmpty(Cell cell) {
switch (cell.getCellType()) {
case STRING:
return "".equals(cell.getStringCellValue().trim());
default:
return false;
}
}
private static void removeColumns(Sheet sheet, List<Integer> colsToDelete) {
for (Row row : sheet) {
for (Integer colIndex : colsToDelete) {
if (row.getCell(colIndex) != null) {
row.removeCell(row.getCell(colIndex));
}
}
}
}
}
```
此代码片段展示了如何读取指定路径下的Excel文档,识别其中完全由空白组成的列,并最终将其从表格结构中剔除[^1]。
阅读全文
相关推荐






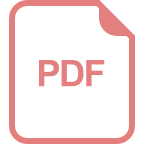










