3d可变形卷积pytorch
时间: 2023-09-19 21:09:29 浏览: 378
对于3D可变形卷积(3D Deformable Convolution)的PyTorch实现,你可以使用`torch.nn.Conv3d`结合自定义的可变形操作来实现。以下是一个简单的示例代码:
```python
import torch
import torch.nn as nn
from torch.autograd import Function
class DeformConv3dFunction(Function):
@staticmethod
def forward(ctx, input, offset, weight, bias=None):
ctx.save_for_backward(input, offset, weight, bias)
output = input.new_empty(DeformConv3dFunction._output_size(input, weight))
# 在这里实现可变形卷积的前向传播操作
# 使用偏移量(offset)来计算采样点的位置,再根据权重(weight)进行卷积运算
return output
@staticmethod
def backward(ctx, grad_output):
input, offset, weight, bias = ctx.saved_tensors
grad_input = grad_offset = grad_weight = grad_bias = None
# 在这里实现可变形卷积的反向传播操作
# 根据grad_output和之前保存的参数,计算梯度
return grad_input, grad_offset, grad_weight, grad_bias
@staticmethod
def _output_size(input, weight):
# 根据输入和权重的形状计算输出的大小
return input.size(0), weight.size(0), input.size(2), input.size(3), input.size(4)
class DeformConv3d(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size, stride=1, padding=0, bias=True):
super(DeformConv3d, self).__init__()
self.offset_conv = nn.Conv3d(in_channels, 3 * kernel_size**3, kernel_size, stride, padding)
self.conv = nn.Conv3d(in_channels, out_channels, kernel_size, stride, padding, bias=bias)
def forward(self, input):
offset = self.offset_conv(input)
output = DeformConv3dFunction.apply(input, offset, self.conv.weight, self.conv.bias)
return output
# 使用示例
input = torch.randn(1, 3, 10, 10, 10)
offset_conv = DeformConv3d(3, 64, 3)
output = offset_conv(input)
```
注意,这只是一个简单的示例代码,实际使用中可能需要根据需求进行适当的修改和调整。希望能对你有所帮助!如果还有其他问题,请继续提问。
阅读全文
相关推荐
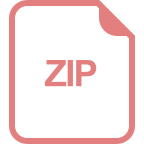
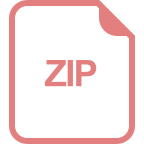
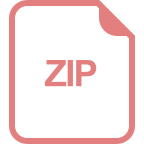
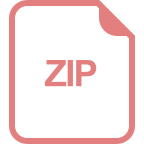
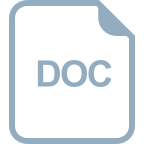
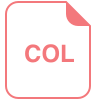
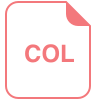
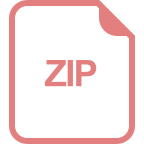
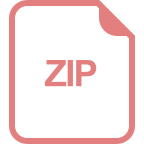