单个结构体成员为1个字符串和1个无符号整数 输入字符串后需要和数组里面每个结构体成员的字符串进行匹配,匹配到后返回对应的无符号整数,如何做查找效率最高。要求,结构体成员个数不确定,可以增加或删除。举例用C语言
时间: 2024-11-06 07:35:58 浏览: 3
为了实现高效查找,你可以考虑动态内存分配和使用哈希表(如`unordered_map`或`std::map`)来存储结构体及其关联的无符号整数。这里以C++为例:
```c++
#include <iostream>
#include <unordered_map>
// 假设我们有一个结构体,其中包含一个字符串和一个无符号整数
struct MyStruct {
std::string str;
uint32_t num;
};
// 动态分配结构体数组,假设结构体数量由`num_structs`变量控制
MyStruct* structs = new MyStruct[num_structs];
// 初始化哈希表,将字符串作为键,无符号整数作为值
std::unordered_map<std::string, uint32_t> hash_table;
// 添加结构体到哈希表
for (int i = 0; i < num_structs; ++i) {
hash_table[strings[i]] = structs[i].num;
}
// 查找功能
uint32_t find_num(const std::string& target_str) const {
auto it = hash_table.find(target_str);
if (it != hash_table.end()) {
return it->second;
}
// 如果未找到,可以选择返回某个默认值或抛异常
return 0; // 返回默认值示例
}
// 示例用法
int main() {
// 初始化结构体和字符串数组
// ...
// 创建并填充哈希表
// ...
std::string input_str = "example_string";
uint32_t result = find_num(input_str);
std::cout << "匹配到的无符号整数: " << result << std::endl;
// 清理内存
delete[] structs;
return 0;
}
```
阅读全文
相关推荐
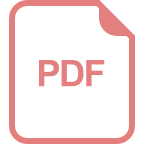
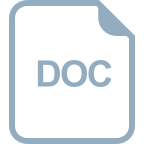
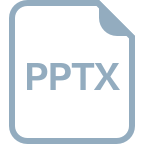

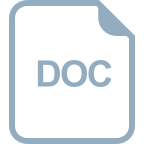
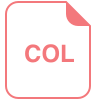
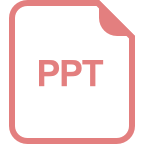
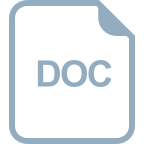
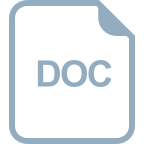
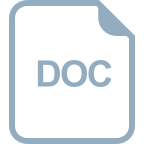
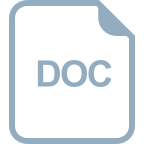
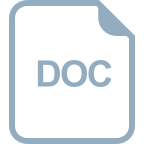
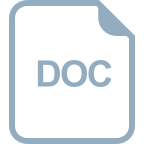
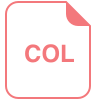
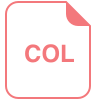


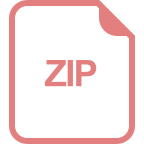