C语言多线程拷贝文件
时间: 2023-11-23 17:56:04 浏览: 60
以下是一个使用C语言多线程拷贝文件的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <pthread.h>
#define BUFFER_SIZE 1024
struct copy_args {
char *src_file;
char *dst_file;
int start_pos;
int end_pos;
};
void *copy_file(void *arg) {
struct copy_args *args = (struct copy_args *)arg;
FILE *src_fp = fopen(args->src_file, "rb");
FILE *dst_fp = fopen(args->dst_file, "wb");
if (src_fp == NULL || dst_fp == NULL) {
printf("Failed to open file.\n");
exit(1);
}
fseek(src_fp, args->start_pos, SEEK_SET);
int len = args->end_pos - args->start_pos + 1;
char buffer[BUFFER_SIZE];
int read_len, write_len;
while (len > 0 && (read_len = fread(buffer, 1, BUFFER_SIZE, src_fp)) > 0) {
if (read_len > len) {
read_len = len;
}
write_len = fwrite(buffer, 1, read_len, dst_fp);
if (write_len != read_len) {
printf("Failed to write file.\n");
exit(1);
}
len -= read_len;
}
fclose(src_fp);
fclose(dst_fp);
return NULL;
}
void multi_thread_copy(char *src_file, char *dst_file, int thread_num) {
FILE *fp = fopen(src_file, "rb");
if (fp == NULL) {
printf("Failed to open file.\n");
exit(1);
}
fseek(fp, 0, SEEK_END);
int file_size = ftell(fp);
fclose(fp);
int block_size = file_size / thread_num;
pthread_t *threads = (pthread_t *)malloc(thread_num * sizeof(pthread_t));
struct copy_args *args = (struct copy_args *)malloc(thread_num * sizeof(struct copy_args));
for (int i = 0; i < thread_num; i++) {
args[i].src_file = src_file;
args[i].dst_file = dst_file;
args[i].start_pos = i * block_size; if (i == thread_num - 1) {
args[i].end_pos = file_size - 1;
} else {
args[i].end_pos = (i + 1) * block_size - 1;
}
pthread_create(&threads[i], NULL, copy_file, &args[i]);
}
for (int i = 0; i < thread_num; i++) {
pthread_join(threads[i], NULL);
}
free(threads);
free(args);
}
int main() {
char *src_file = "/home/XXX";
char *dst_file = "/home/dir/XXX";
int thread_num = 4;
multi_thread_copy(src_file, dst_file, thread_num);
printf("Copy file finished.\n");
return 0;
}
```
相关推荐
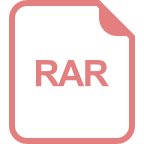
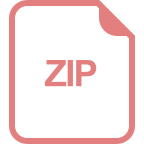
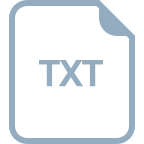
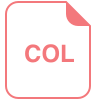
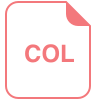












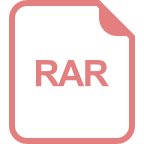